Java发送form-data格式的请求上传multipart文件
时间: 2024-09-27 18:15:48 浏览: 38
在Java中,特别是使用了如Spring框架这样的工具,发送包含multipart文件的form-data格式请求通常涉及到`HttpURLConnection`、`HttpClient`或者第三方库如Apache HttpClient或OkHttp。这里是一个基本的例子,使用`UrlConnection`:
```java
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.URL;
public class FileUploadExample {
private static final String API_URL = "http://example.com/upload";
public static void main(String[] args) {
try {
// 打开文件流
FileInputStream fileInputStream = new FileInputStream(new File("path_to_your_file"));
// 创建字节数组输出流
ByteArrayOutputStream byteOutStream = new ByteArrayOutputStream();
// 读取并写入文件内容到字节数组
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = fileInputStream.read(buffer)) != -1) {
byteOutStream.write(buffer, 0, bytesRead);
}
fileInputStream.close(); // 关闭输入流
// 设置POST请求
URL url = new URL(API_URL);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW");
// 添加分隔符
byte[] boundary = "--" + "----WebKitFormBoundary7MA4YWxkTrZu0gW".getBytes();
connection.getOutputStream().write(boundary);
// 发送二进制数据
connection.getOutputStream().write(byteOutStream.toByteArray());
// 添加文件结束标记
byte[] endBoundary = new byte[] {'\r', '\n', '--' + '----WebKitFormBoundary7MA4YWxkTrZu0gW--'};
connection.getOutputStream().write(endBoundary);
// 获取响应
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
System.out.println("File uploaded successfully.");
} else {
System.err.println("Failed to upload file. Response code: " + responseCode);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
阅读全文
相关推荐
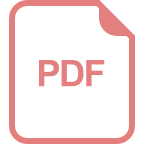
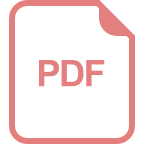
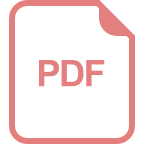















