定义数组,存放5个学生的成绩【成绩值自己设定】,将成绩从大到小排序,获得成绩之和,平均成绩,最小成绩,最大成绩。Java
时间: 2024-12-06 16:26:59 浏览: 17
在Java中,我们可以定义一个名为`StudentScore`的对象数组来存储每个学生的成绩,并使用`Arrays.sort`函数对成绩进行降序排列。以下是详细的步骤:
首先,定义一个`StudentScore`类,包含姓名和成绩属性:
```java
class StudentScore {
String name;
double score;
// 构造函数、getters和setters省略
}
```
然后,创建并填充数组:
```java
public class Main {
public static void main(String[] args) {
StudentScore[] students = new StudentScore[5];
students[0] = new StudentScore("张三", 90.0);
students[1] = new StudentScore("李四", 85.0);
students[2] = new StudentScore("王五", 95.0);
students[3] = new StudentScore("赵六", 75.0);
students[4] = new StudentScore("孙七", 88.0);
// 排序并计算成绩相关统计
sortAndCalculate(students);
}
public static void sortAndCalculate(StudentScore[] scores) {
Arrays.sort(scores, Comparator.comparingDouble(StudentScore::getScore).reversed());
double totalScore = 0.0;
double sumScores = 0.0;
double minScore = Double.MAX_VALUE;
double maxScore = Double.MIN_VALUE;
for (StudentScore student : scores) {
totalScore += student.score;
sumScores += student.score;
minScore = Math.min(minScore, student.score);
maxScore = Math.max(maxScore, student.score);
}
double averageScore = totalScore / scores.length;
System.out.println("Sorted Scores:");
for (StudentScore student : scores) {
System.out.println(student.name + ": " + student.score);
}
System.out.println("Total Score: " + totalScore);
System.out.println("Average Score: " + averageScore);
System.out.println("Minimum Score: " + minScore);
System.out.println("Maximum Score: " + maxScore);
}
}
```
这段代码首先对成绩数组按降序排序,然后分别累加总分,求和,以及找到最小和最大的成绩。最后计算平均分并打印所有统计结果。
阅读全文
相关推荐
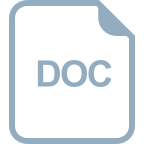
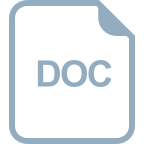
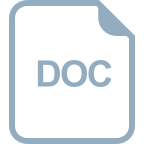












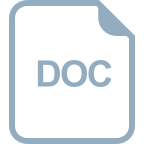
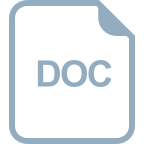
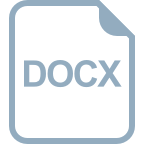
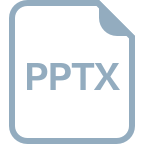