货郎担算法和c++代码及具体解释
时间: 2024-12-27 21:32:53 浏览: 3
货郎担算法,又称为旅行商问题(Traveling Salesman Problem,TSP)的启发式搜索解法之一,是一个经典的组合优化问题。该算法试图找到从一组给定的城市出发,访问每个城市恰好一次,并返回原点的最短路径。货郎担算法的核心思想是利用贪心策略,每次选择当前未访问城市的最近邻居作为下一站。
虽然不是严格的最优解,但在实际应用中可以得到接近最优的结果,尤其是在解决大规模问题时。以下是简单的 C++ 代码实现:
```cpp
#include <iostream>
#include <vector>
#include <limits>
// 定义城市类,包含坐标信息
class City {
public:
int x, y;
City(int _x, int _y) : x(_x), y(_y) {}
};
// 计算两点之间的距离
int distance(City& a, City& b) {
return std::abs(a.x - b.x) + std::abs(a.y - b.y);
}
// 货郎担算法
std::vector<int> huckel_tsp(std::vector<City>& cities) {
// 初始化
int n = cities.size();
std::vector<std::vector<int>> dist(n, std::vector<int>(n, 0));
for (size_t i = 0; i < n; ++i) {
for (size_t j = i + 1; j < n; ++j) {
dist[i][j] = dist[j][i] = distance(cities[i], cities[j]);
}
}
// 选择第一个城市作为起始点
std::vector<int> tour(1, 0);
while (tour.size() != n) {
// 找到剩余城市中最远的一个并添加
int farthest_idx = 0;
for (size_t i = 1; i < n; ++i) {
if (dist[tour.back()][i] > dist[tour.back()][farthest_idx]) {
farthest_idx = i;
}
}
tour.push_back(farthest_idx);
}
tour.pop_back(); // 回到起点
return tour;
}
int main() {
std::vector<City> cities = {City(0, 0), City(1, 4), City(5, 6), City(3, 8)};
std::vector<int> tour = huckel_tsp(cities);
for (int city_id : tour) {
std::cout << "City " << city_id << ": (" << cities[city_id].x << ", "
<< cities[city_id].y << ")" << std::endl;
}
return 0;
}
```
这个程序首先计算出所有城市间的距离矩阵,然后通过迭代添加最远处的城市形成路径,直到遍历完所有的城市。注意这只是一个简化的版本,实际应用中可能需要加入随机化或其他改进。
阅读全文
相关推荐
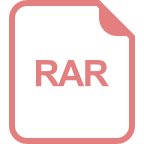
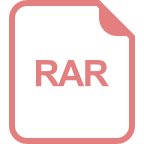
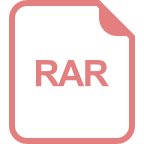
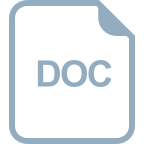
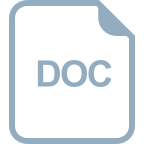

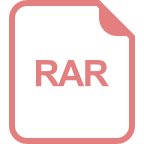
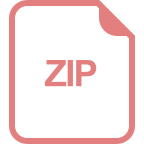
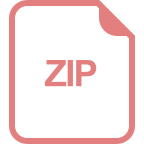
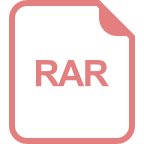
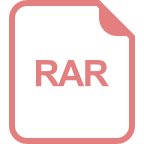
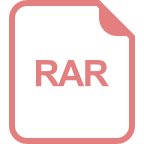
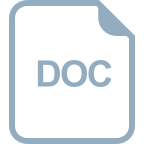
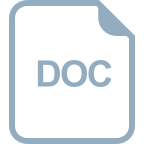
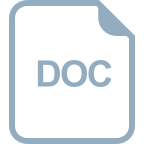
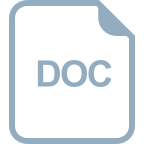
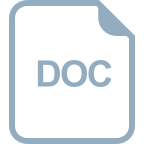
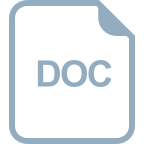
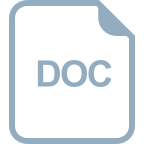