牛顿插值法的Python实现,有图像显示
时间: 2024-10-18 21:11:32 浏览: 44
牛顿插值法是一种数值分析方法,用于估算函数在某点的近似值,特别是在数据密集的情况下。在Python中,我们可以使用`numpy`库结合`matplotlib`库来展示这个过程。以下是简单的三阶多项式插值的一个例子:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义数据点
x_data = np.array([0, 1, 2, 3])
y_data = np.array([2, 4, 6, 8])
# 计算插值系数
def newton_interpolation(x, y):
n = len(y) - 1
a = np.zeros((n+1, n+1))
b = np.zeros(n+1)
for i in range(n+1):
for j in range(i, n+1):
a[i][j] = factorial(j) / (factorial(i)*factorial(j-i))
b[i] = y[i]
coeffs = np.linalg.solve(a, b)
return coeffs
# 计算并存储插值多项式的系数
coeffs = newton_interpolation(x_data, y_data)
# 定义插值函数
def interpolate_poly(x, coeffs):
result = 0
for i in range(len(coeffs)):
result += coeffs[i]*x**(i+1)
return result
# 插值计算并绘制图表
x_values = np.linspace(min(x_data), max(x_data), 100)
y_interpolated = interpolate_poly(x_values, coeffs)
plt.scatter(x_data, y_data, label='Data Points')
plt.plot(x_values, y_interpolated, 'r', label='Interpolated Curve')
plt.xlabel('X')
plt.ylabel('Y')
plt.legend()
plt.show()
# 辅助函数计算阶乘
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
阅读全文
相关推荐
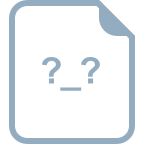
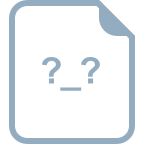
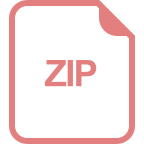


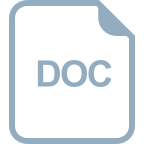
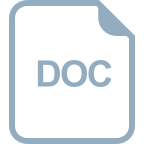
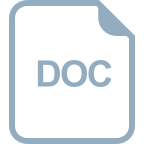
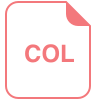





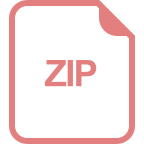
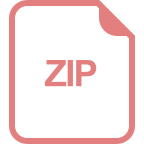
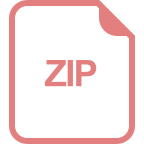