用C++编写一个单链表,功能包含插入,查找,删除,合并,打印
时间: 2024-11-13 13:27:29 浏览: 2
在C++中,我们可以创建一个简单的单链表结构,它包含了节点类(Node)和链表类(LinkedList)。以下是基本的操作实现:
首先,我们定义一个Node类,用于存储数据和指向下一个节点的指针:
```cpp
class Node {
public:
int data;
Node* next;
Node(int value) : data(value), next(nullptr) {}
};
```
然后,我们创建LinkedList类,包含插入、查找、删除、合并和打印函数:
```cpp
class LinkedList {
private:
Node* head; // 链表头节点
public:
LinkedList() : head(nullptr) {}
// 插入元素到链表尾部
void insert(int value) {
if (!head) {
head = new Node(value);
} else {
Node* temp = head;
while (temp->next) {
temp = temp->next;
}
temp->next = new Node(value);
}
}
// 查找元素
bool search(int value) const {
Node* temp = head;
while (temp) {
if (temp->data == value) {
return true;
}
temp = temp->next;
}
return false;
}
// 删除元素
void remove(int value) {
if (!head || !head->data) {
return;
}
if (head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* current = head;
while (current->next && current->next->data != value) {
current = current->next;
}
if (current->next) {
current->next = current->next->next;
delete current->next;
}
}
// 合并两个有序链表
LinkedList merge(LinkedList& otherList) {
LinkedList mergedList;
Node* current1 = head;
Node* current2 = otherList.head;
while (current1 && current2) {
if (current1->data < current2->data) {
mergedList.insert(current1->data);
current1 = current1->next;
} else {
mergedList.insert(current2->data);
current2 = current2->next;
}
}
// 将剩余部分添加到结果
while (current1) {
mergedList.insert(current1->data);
current1 = current1->next;
}
while (current2) {
mergedList.insert(current2->data);
current2 = current2->next;
}
return mergedList;
}
// 打印链表
void print() const {
Node* temp = head;
while (temp) {
std::cout << temp->data << " -> ";
temp = temp->next;
}
std::cout << "nullptr" << std::endl;
}
};
```
以上就是C++中实现单链表的基本操作。你可以根据需要在程序中实例化这个LinkedList,并使用这些方法。
阅读全文
相关推荐
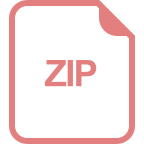
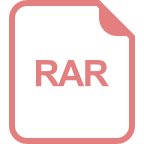


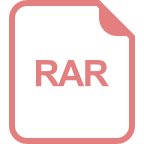
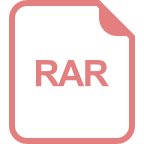
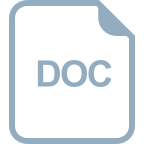
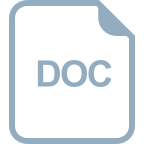

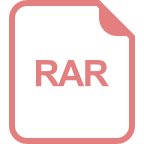
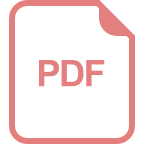
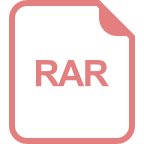
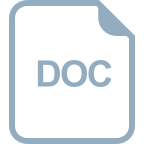
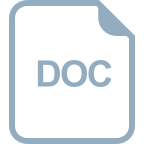
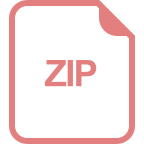
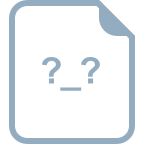
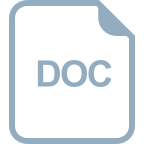