使用c++实现上述算法
时间: 2024-10-07 08:02:08 浏览: 23
在C++中实现这个三路快速选择算法寻找数组最大值和最小值的过程如下:
```cpp
#include <vector>
using namespace std;
// 用于三路划分找到最小值
int partition(vector<int>& arr, int low, int high) {
int pivot = arr[(low + high) / 2];
while (true) {
while (arr[low] < pivot) low++;
while (arr[high] > pivot) high--;
if (low <= high)
swap(arr[low], arr[high]);
else
return high;
}
}
// 三路快速选择函数
pair<int, int> quickSelect(vector<int>& arr, int low, int high, bool findMax) {
if (low == high)
return make_pair(arr[low], arr[low]); // 单元素的情况
int pivotIndex = partition(arr, low, high);
if (pivotIndex == high || !findMax)
return make_pair(arr[pivotIndex], arr[low]); // 返回最大值或最小值
return quickSelect(arr, low, pivotIndex - 1, findMax) ||
quickSelect(arr, pivotIndex + 1, high, findMax); // 递归处理剩余区间
}
// 主函数
void findMinMax(const vector<int>& arr) {
int n = arr.size();
pair<int, int> result = quickSelect(arr, 0, n - 1, false); // 找最小值
cout << "Minimum value: " << result.first << endl;
result = quickSelect(arr, 0, n - 1, true); // 调整为findMax找最大值
cout << "Maximum value: " << result.second << endl;
}
```
在这个代码中,`partition` 函数完成了三路划分,`quickSelect` 递归地调用自身,根据需求判断是在左边还是右边继续搜索。`findMinMax` 函数则是主入口,调用两次 `quickSelect` 分别求解最小值和最大值。
阅读全文
相关推荐
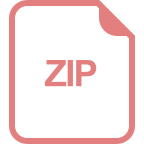
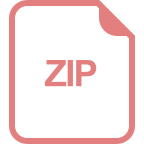
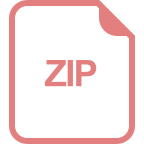
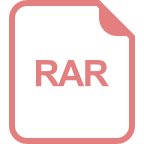
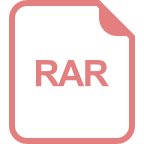
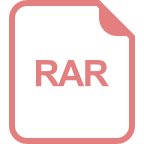
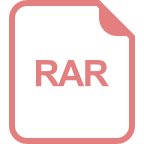
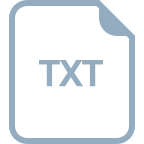
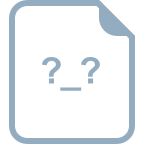
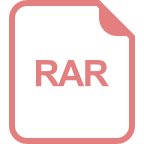
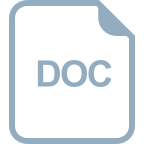
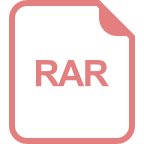
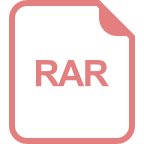
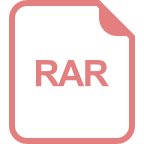
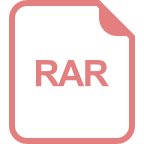
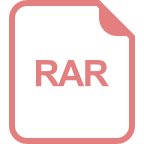
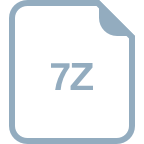
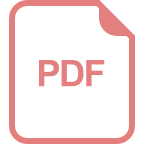
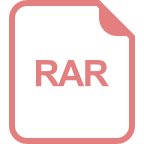