android 上传文件到服务器
时间: 2023-08-26 18:05:49 浏览: 37
要在Android应用中将文件上传到服务器,可以按照以下步骤进行操作:
1. 首先,确保应用的AndroidManifest.xml文件中添加了以下权限:
```xml
<uses-permission android:name="android.permission.INTERNET" />
```
这样才能进行网络通信。
2. 创建一个HTTP请求来上传文件。可以使用Java的HttpURLConnection类或者第三方库如OkHttp或Volley来实现。以下是使用HttpURLConnection的示例代码:
```java
URL url = new URL("http://example.com/upload"); // 服务器的上传地址
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setDoOutput(true);
connection.setRequestMethod("POST");
// 设置请求头
connection.setRequestProperty("Content-Type", "multipart/form-data;boundary=" + boundary);
// 创建请求体
DataOutputStream outputStream = new DataOutputStream(connection.getOutputStream());
outputStream.writeBytes("--" + boundary + "\r\n");
outputStream.writeBytes("Content-Disposition: form-data; name=\"file\";filename=\"" + fileName + "\"" + "\r\n");
outputStream.writeBytes("Content-Type: " + mimeType + "\r\n\r\n");
// 将文件数据写入请求体
File file = new File(filePath);
FileInputStream fileInputStream = new FileInputStream(file);
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = fileInputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
outputStream.writeBytes("\r\n");
outputStream.writeBytes("--" + boundary + "--\r\n");
// 关闭流
fileInputStream.close();
outputStream.flush();
outputStream.close();
// 获取服务器响应
int responseCode = connection.getResponseCode();
```
在上面的代码中,你需要修改以下变量:
- `url`:服务器的上传地址。
- `boundary`:用于分隔请求体中不同部分的边界字符串。
- `fileName`:要上传的文件名。
- `mimeType`:文件的MIME类型。
- `filePath`:要上传的文件的本地路径。
3. 处理服务器的响应。可以根据`responseCode`来判断上传是否成功,以及根据需要处理服务器返回的数据。
请注意,上述代码仅为示例,实际实现中可能需要根据你的服务器端要求进行一些调整。另外,为了更好的用户体验,可以将文件上传操作放在后台线程中执行,以避免阻塞主线程。
相关推荐
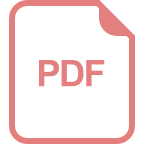
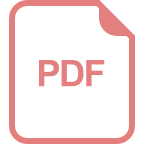
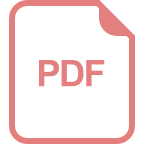
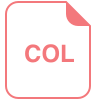
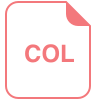
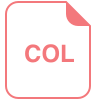
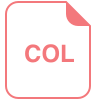










