用Python随机生成9*9迷宫,绘制出9*9迷宫形状,墙壁为黑,其中有一条可走路线为红
时间: 2024-10-09 17:04:37 浏览: 24
在Python中,我们可以使用`numpy`库创建一个二维数组来模拟迷宫,然后利用matplotlib库进行可视化。这里是一个简单的例子:
首先,你需要安装必要的库,如果尚未安装,可以使用pip命令安装:
```bash
pip install numpy matplotlib
```
接下来,我们可以编写代码生成迷宫和绘制迷宫:
```python
import numpy as np
import matplotlib.pyplot as plt
# 创建一个9x9的二维数组,初始化所有元素为墙壁(0表示空地,1表示墙壁)
maze = np.zeros((9, 9), dtype=int)
# 设置起点和终点位置(这里仅设置一条路径,你可以根据需要调整)
start = (0, 0)
end = (8, 8)
# 使用深度优先搜索算法生成迷宫
def dfs(maze, x, y):
if x < 0 or y < 0 or x >= maze.shape[0] or y >= maze.shape[1] or maze[x, y] != 0:
return
maze[x, y] = 1
dfs(maze, x+1, y) # 右
dfs(maze, x-1, y) # 左
dfs(maze, x, y+1) # 下
dfs(maze, x, y-1) # 上
dfs(maze, start[0], start[1])
# 将起点和终点标记为红色(1代表道路,0代表墙壁)
maze[start] = maze[end] = 1
# 绘制迷宫
plt.imshow(maze, cmap='gray', interpolation='nearest')
plt.plot(*np.where(maze == 1), 'r-', linewidth=2) # 红色路径
plt.scatter(start[0], start[1], c='red') # 起点
plt.scatter(end[0], end[1], c='red') # 终点
plt.xticks(np.arange(9), [], fontsize=0)
plt.yticks(np.arange(9), [], fontsize=0)
plt.title('9x9迷宫')
plt.show()
```
在这个代码中,我们首先创建一个全0的9x9矩阵作为迷宫,然后从起点开始,通过深度优先搜索算法逐步开辟路径,并将路径区域标记为1。最后,使用matplotlib绘制迷宫,红色线条表示路径。
相关推荐
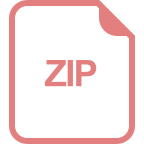
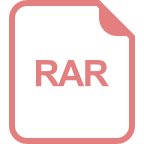
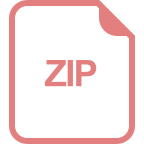














