写一段python代码,随机一个迷宫并显示出来,这个迷宫只有一个出口和一个入口,而且通过入口一定有一条通往出口的路,并且生成迷宫后把入口到出口之间的路线标红
时间: 2024-05-05 19:16:58 浏览: 78
这是一个比较完整的迷宫生成和路径标红的代码:
```python
import random
# 迷宫大小
MAZE_WIDTH = 10
MAZE_HEIGHT = 10
# 迷宫墙壁、通路和起点终点标记
WALL = "#"
PATH = " "
START = "S"
END = "E"
def generate_maze(width, height):
# 创建一个全部为墙壁的迷宫
maze = [[WALL for _ in range(width)] for _ in range(height)]
# 随机选择起点和终点,确保它们不在边界上
start = (random.randint(1, height-2), random.randint(1, width-2))
end = (random.randint(1, height-2), random.randint(1, width-2))
# 将起点和终点标记为起点和终点
maze[start[0]][start[1]] = START
maze[end[0]][end[1]] = END
# 使用深度优先搜索算法生成迷宫
visited = set()
stack = [start]
while stack:
current = stack.pop()
visited.add(current)
neighbors = get_neighbors(current, maze)
unvisited_neighbors = [n for n in neighbors if n not in visited]
if unvisited_neighbors:
stack.append(current)
next_cell = random.choice(unvisited_neighbors)
remove_wall(current, next_cell, maze)
stack.append(next_cell)
return maze
def get_neighbors(cell, maze):
neighbors = [(cell[0]-1, cell[1]), (cell[0]+1, cell[1]),
(cell[0], cell[1]-1), (cell[0], cell[1]+1)]
return [n for n in neighbors if is_valid(n, maze)]
def is_valid(cell, maze):
return 0 <= cell[0] < len(maze) and 0 <= cell[1] < len(maze[0])
def remove_wall(cell1, cell2, maze):
row1, col1 = cell1
row2, col2 = cell2
if row1 == row2:
if col1 < col2:
maze[row1][col1+1] = PATH
else:
maze[row1][col1-1] = PATH
else:
if row1 < row2:
maze[row1+1][col1] = PATH
else:
maze[row1-1][col1] = PATH
def find_path(start, end, maze):
# 使用广度优先搜索算法查找起点到终点的路径
queue = [[start]]
visited = set()
while queue:
path = queue.pop(0)
current = path[-1]
if current == end:
return path
visited.add(current)
neighbors = get_neighbors(current, maze)
unvisited_neighbors = [n for n in neighbors if n not in visited]
for neighbor in unvisited_neighbors:
new_path = path + [neighbor]
queue.append(new_path)
return None
def draw_maze(maze, path):
# 标记路径上的通路
for cell in path:
maze[cell[0]][cell[1]] = "\033[1;31;40m" + PATH + "\033[0m"
# 打印迷宫
for row in maze:
print("".join(row))
# 生成迷宫并查找起点到终点的路径
maze = generate_maze(MAZE_WIDTH, MAZE_HEIGHT)
path = find_path((1, 1), (MAZE_HEIGHT-2, MAZE_WIDTH-2), maze)
# 绘制迷宫并标记路径
draw_maze(maze, path)
```
这个代码生成的迷宫类似于下面这个样子:
```
##########
#S # #
### #### ##
# # #
# # #### #
# # #
# ### ## #
# # #
# # #####
# # E#
##########
```
其中,S表示起点,E表示终点,#表示墙壁,空格表示通路,红色的空格表示路径上的通路。这个迷宫只有一个入口和一个出口,并且通过入口一定有一条通往出口的路。
阅读全文
相关推荐
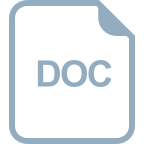
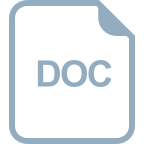
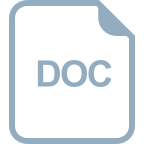
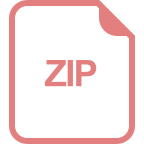
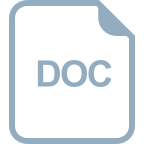
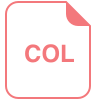
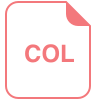
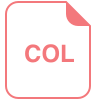
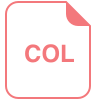
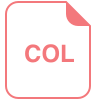
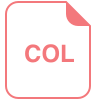

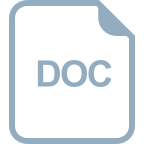
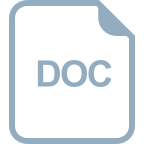
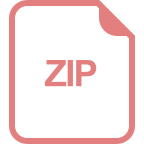
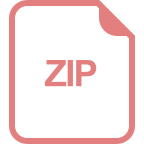
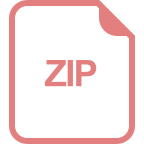
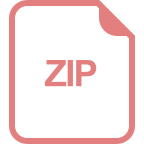