``` #include<stdio.h> int main(void) { char str [100]; scanf_s("%99s", str); printf("%s", str); return 0; }```当输入内容时,程序崩溃了
时间: 2024-11-17 14:28:43 浏览: 3
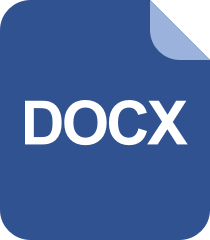
C语言程序设计常见上机实验题目汇总.docx
这段C语言代码尝试通过`scanf_s`函数从用户那里读取最多99个字符到`str`数组中,然后打印这个字符串。然而,存在两个潜在问题可能导致程序崩溃:
1. **内存溢出**:`scanf_s` 函数在读入字符串前没有检查剩余空间。如果用户输入超过99个字符(包括空格),`str`数组可能会越界,从而覆盖其他未初始化的数据,引发运行时错误。
2. **宽字符函数**:`scanf_s` 是Windows平台特定的宽字符版本,如果你在非Windows系统上运行这段代码,它可能不会正常工作。对于标准版的`scanf`,你需要使用`scanf`而非`scanf_s`。
为了避免崩溃,你可以做以下修改:
1. **动态分配内存**:使用`fgets`代替`scanf`,可以指定包含终止符`\n`在内的最大字符数,如`fgets(str, sizeof(str), stdin)`。
2. **检查输入长度**:在接收输入后,检查实际读取的字符数是否在范围内。
修复后的代码可能像这样:
```c
#include<stdio.h>
#define MAX_LEN 100
int main(void)
{
char str[MAX_LEN];
size_t len;
// fgets allows for newline character and limits input to MAX_LEN - 1
if (fgets(str, MAX_LEN, stdin)) {
str[strlen(str) - 1] = '\0'; // Remove the newline if present
len = strlen(str); // Get the actual length of the string
if (len <= MAX_LEN - 1) { // Check if not overflowed
printf("%s", str);
} else {
printf("Input too long!\n");
}
}
return 0;
}
```
阅读全文
相关推荐
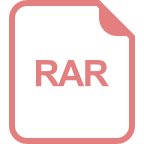
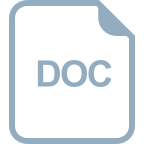















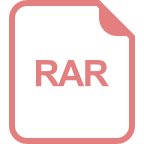
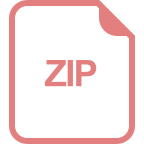