在一个 n×m的矩阵中,有一个数字出现了超过一半的次数,请设计一个高效算法找到这个数字。 输入格式 输入第一行包含两个整数 n 和 m,表示矩阵的大小 (1≤n,m≤103),用C语言写出
时间: 2024-11-04 14:15:24 浏览: 23
这是一个经典的统计学问题,可以使用哈希表来解决。这里是一个简化的C语言算法描述:
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX_SIZE 1001
// 哈希表存储元素及其计数
typedef struct {
int num;
int count;
} HashTable;
// 遍历矩阵并更新哈希表
void updateHashTable(int matrix[MAX_SIZE][MAX_SIZE], HashTable* hashTable, int n, int m) {
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
if (hashTable[matrix[i][j]].count == 0) {
hashTable[matrix[i][j]].num = matrix[i][j];
}
hashTable[matrix[i][j]].count++;
}
}
}
// 查找出现次数超过一半的数字
int majorityElement(int matrix[MAX_SIZE][MAX_SIZE], int n, int m) {
HashTable hashTable[MAX_SIZE * MAX_SIZE + 1];
updateHashTable(matrix, hashTable, n, m);
int candidate = -1;
for (int i = 0; i <= MAX_SIZE * MAX_SIZE; i++) {
if (hashTable[i].count > n / 2) {
if (candidate == -1 || hashTable[candidate].count < hashTable[i].count) {
candidate = i;
}
}
}
return candidate;
}
int main() {
int n, m;
scanf("%d %d", &n, &m);
int matrix[n][m]; // 假设已经读取了矩阵内容
int result = majorityElement(matrix, n, m);
printf("The number that appears more than half of the time is: %d\n", result);
return 0;
}
```
注意,这个算法的时间复杂度是O(n*m),其中n和m是矩阵的维度,因为它需要遍历整个矩阵。空间复杂度是O(m*n),因为哈希表的大小取决于矩阵的大小。
阅读全文
相关推荐
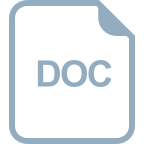
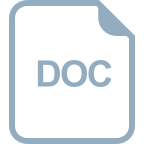
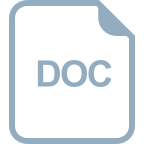

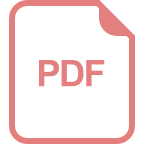
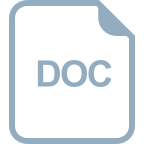
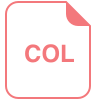
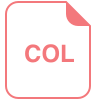
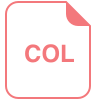
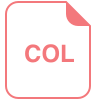
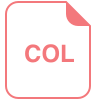
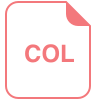
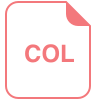
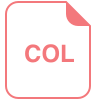
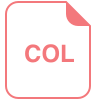
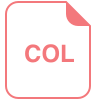
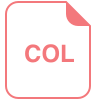
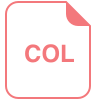