假设用户可能使用的输入仅包含小写字母a~z和空格,请编写一个程序,对输入字符串进行凯撒密码加密,直接输出结果,其中空格不用进行加密处理。使用input()获得输入。
时间: 2024-12-05 11:15:36 浏览: 40
在Python中,我们可以创建一个简单的凯撒密码加密函数,只对字母字符进行位移操作,并保持空格不变。以下是一个示例程序:
```python
def caesar_cipher_encrypt(input_string, shift):
encrypted_string = ""
for char in input_string:
if char.isalpha(): # 检查是否为字母
shifted_char = chr((ord(char.lower()) - ord('a') + shift) % 26 + ord('a')) # 加密字母字符
encrypted_string += shifted_char
else:
encrypted_string += char # 保留空格和其他非字母字符
return encrypted_string
# 获取用户输入并转换为小写
user_input = input().lower()
# 设置加密位移量(例如:3表示向右移动3个位置)
shift_amount = 3
# 进行加密
encrypted_text = caesar_cipher_encrypt(user_input, shift_amount)
print("Encrypted text:", encrypted_text)
相关问题
Python假设用户可能使用的输入仅包含小写字母a~z和空格,请编写一个程序,对输入字符串进行凯撒密码加密,直接输出结果,其中空格不用进行加密处理。使用input()获得输入。
shift = 3
plaintext = input("请输入需要加密的字符串:")
ciphertext = ""
for c in plaintext:
if c == " ":
ciphertext += " "
else:
ascii_code = ord(c)
shifted_ascii_code = ((ascii_code - 97) + shift) % 26 + 97
ciphertext += chr(shifted_ascii_code)
print("加密后的字符串为:", ciphertext)
假设用户可能使用的输入仅包含小写字母a~z和空格,请用python编写一个程序,对输入字符串进行凯撒密码加密,直接输出结果,其中空格不用进行加密处理。使用input()获得输入。
以下是代码实现:
```python
plain_text = input("请输入要加密的字符串:")
shift = int(input("请输入加密位移(1~25):"))
cipher_text = ""
for char in plain_text:
if char != " ":
# 将小写字母转换为 0~25 的数字,加上位移后再转换为字母
cipher_text += chr((ord(char) - 97 + shift) % 26 + 97)
else:
cipher_text += " "
print("加密后的字符串为:", cipher_text)
```
首先通过 `input()` 获取用户输入的明文和加密位移,然后遍历字符串中的每个字符,如果是空格则不进行加密,否则将小写字母转换为 0~25 的数字,加上位移后再转换为字母,最后将加密后的字符拼接到密文字符串中。最后输出密文。
阅读全文
相关推荐
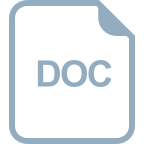
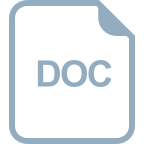
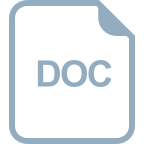




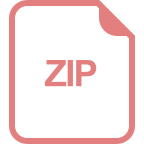








