要求实现以下功能: 顺序存储结构线性表的基本操作 (1)生成长度为8的线性表。 (2)查找数据。 (3)选择插入数据。 (4)删除数据。
时间: 2024-11-05 17:32:20 浏览: 9
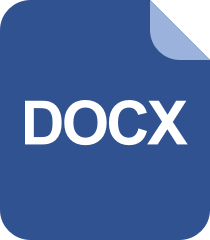
《数据结构C++版》实验一:线性表的顺序存储结构实验报告
在顺序存储结构(如数组或链表)中实现线性表的基本操作,你需要考虑以下几个步骤:
1. **生成长度为8的线性表**:
```python
linear_list = [None] * 8 # 使用默认值创建一个空的顺序表,如果需要其他值填充,可以用指定的值替换None
```
2. **查找数据**:
- 对于数组(索引访问),可以使用下标操作:
```python
def search(data, target):
for i in range(len(linear_list)):
if linear_list[i] == target:
return i
return -1 # 如果未找到目标,返回-1表示不存在
```
- 对于链表(需遍历节点),查找时间复杂度较高,可能需要迭代整个链表。
3. **选择插入数据**:
- 数组中插入:
```python
def insert(data, index, value):
if index < 0 or index > len(linear_list):
raise IndexError
linear_list.insert(index, value)
```
- 链表中插入(例如单链表):
```python
class Node:
def __init__(self, data=None, next=None):
self.data = data
self.next = next
def insert_node_at_head(head, value):
new_node = Node(value)
new_node.next = head
return new_node
```
4. **删除数据**:
- 数组中删除:
```python
def delete(data, index):
if index < 0 or index >= len(linear_list):
raise IndexError
linear_list.pop(index)
```
- 链表中删除(例如单链表):
```python
def delete_node(head, value):
current = head
prev = None
while current is not None and current.data != value:
prev = current
current = current.next
if current is not None:
prev.next = current.next
```
以上代码示例是针对数组的,对于链表,可能需要修改部分细节。注意在实际应用中,还需要处理边界条件和异常情况。
阅读全文
相关推荐
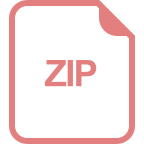
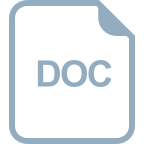















