java通过自定义注解获取方法中被try catch的异常
时间: 2024-10-13 10:07:52 浏览: 31
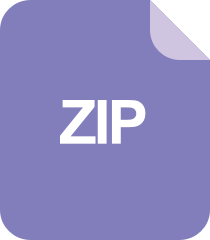
java自定义注解 示例原代码
在Java中,自定义注解用于标记特定元素,如方法、变量等,但它们本身并不能直接获取方法中的异常处理(即try-catch块)。不过,你可以通过反射API来间接实现这个功能。
首先,创建一个自定义注解,例如:
```java
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface CustomCatchAnnotation {
Class<? extends Exception>[] exceptions() default {};
}
```
然后,在需要标注的try-catch方法上使用这个注解:
```java
@CustomCatchAnnotation(exceptions = {IOException.class})
public void process() throws IOException {
try {
// 你的代码...
} catch (IOException e) {
// 异常处理
}
}
```
接着,你可以编写一个工具类,利用`Method.getAnnotation()`方法和反射来检查并提取异常信息:
```java
import java.lang.reflect.Method;
public class AnnotationUtils {
public static List<Class<? extends Exception>> getCaughtExceptions(Method method) {
CustomCatchAnnotation annotation = method.getAnnotation(CustomCatchAnnotation.class);
if (annotation != null) {
return Arrays.asList(annotation.exceptions());
} else {
return Collections.emptyList();
}
}
public static void main(String[] args) {
Method method = YourClass.class.getMethod("process");
List<Class<? extends Exception>> exceptions = getCaughtExceptions(method);
for (Class<? extends Exception> exception : exceptions) {
System.out.println(exception.getName()); // 输出catch到的异常类型
}
}
}
```
这样,你就可以通过`AnnotationUtils.getCaughtExceptions()`方法获取指定方法中自定义注解所声明的异常列表了。
阅读全文
相关推荐
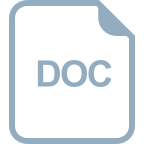
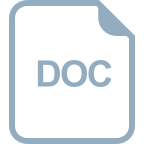
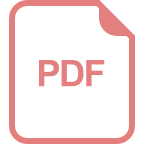
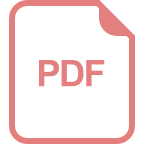
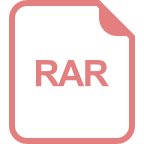
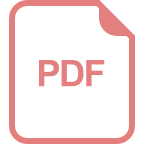
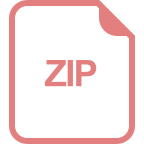
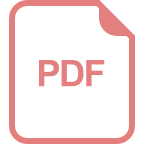
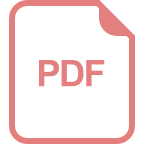
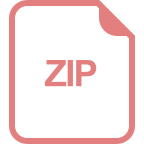
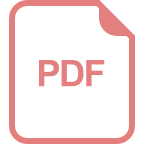
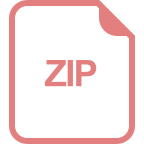
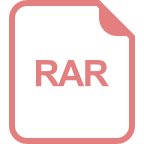
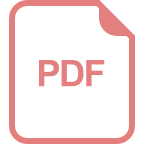
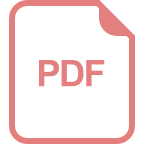
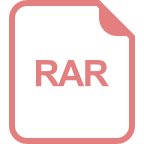
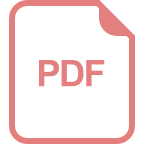
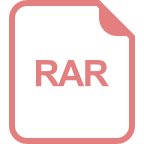