这段代码怎样根据螺栓位置和点云图得到螺栓在相机坐标系下的坐标
时间: 2024-12-15 08:19:02 浏览: 4
该代码通过以下步骤从点云数据中获取螺栓在相机坐标系下的坐标:
1. **初始化**:
- 初始化过程中,移除显示中的旧点云并添加新的点云数据。
2. **计算单个螺栓的位姿**:
- `ClassRansac::ComputeOneBolt` 方法对单个螺栓的点云数据进行处理。
- 使用均匀滤波器 `pcl::UniformSampling` 对点云进行滤波,减少点的数量。
- 使用 RANSAC 算法拟合平面,提取内点并计算质心。
- 质心计算使用 `pcl::compute3DCentroid` 函数。
- 获取平面的法向量,并将其规范化以确保方向一致。
- 最终输出质心和法向量。
3. **计算所有螺栓的位姿**:
- `ClassRansac::ComputeAllBolt` 方法遍历所有螺栓的点云数据,调用 `ComputeOneBolt` 方法计算每个螺栓的位姿。
4. **显示结果**:
- `ClassRansac::DisplayAllPose` 方法启动可视化窗口,显示所有螺栓的位姿。
具体步骤如下:
### 详细步骤
1. **初始化**:
```cpp
void ClassRansac::Init(vector<pcl::PointCloud<pcl::PointXYZ>::Ptr> _VecBoltPointCloud, pcl::PointCloud<pcl::PointXYZ>::Ptr _ALLPointCloud) {
VecBoltPointCloud = _VecBoltPointCloud;
ALLPointCloud = _ALLPointCloud;
BoltNum = VecBoltPointCloud.size();
centroid.resize(BoltNum);
coefficient.resize(BoltNum);
VecViewer[0]->removeAllPointClouds();
VecViewer[0]->removeAllShapes();
VecViewer[0]->addPointCloud<pcl::PointXYZ>(ALLPointCloud, "cloud");
VecViewer[0]->setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_COLOR, 1, 1, 1, "cloud");
VecViewer[0]->setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_POINT_SIZE, 1, "cloud");
}
```
2. **计算单个螺栓的位姿**:
```cpp
void ClassRansac::ComputeOneBolt(pcl::PointCloud<pcl::PointXYZ>::Ptr cloud, Eigen::Vector4f ¢roid, Eigen::VectorXf &coefficient, pcl::visualization::PCLVisualizer::Ptr &viewer) {
pcl::PointCloud<pcl::PointXYZ>::Ptr BoltCloudFiltered(new pcl::PointCloud<pcl::PointXYZ>);
pcl::UniformSampling<pcl::PointXYZ> US;
US.setInputCloud(cloud);
US.setRadiusSearch(0.01);
US.filter(*BoltCloudFiltered);
pcl::SampleConsensusModelPlane<pcl::PointXYZ>::Ptr model_plane(new pcl::SampleConsensusModelPlane<pcl::PointXYZ>(BoltCloudFiltered));
pcl::RandomSampleConsensus<pcl::PointXYZ> ransac(model_plane);
ransac.setDistanceThreshold(0.2);
ransac.computeModel();
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud_plane(new pcl::PointCloud<pcl::PointXYZ>);
vector<int> inliers;
ransac.getInliers(inliers);
pcl::copyPointCloud<pcl::PointXYZ>(*BoltCloudFiltered, inliers, *cloud_plane);
pcl::compute3DCentroid(*cloud_plane, centroid);
cout << "质心:" << centroid[0] << "," << centroid[1] << "," << centroid[2] << endl;
ransac.getModelCoefficients(coefficient);
if (coefficient[3] > 0) {
for (int i = 0; i < 4; ++i) coefficient[i] = -coefficient[i];
}
cout << "法向量:" << coefficient[0] << "," << coefficient[1] << "," << coefficient[2] << endl;
pcl::PointXYZ P1(centroid[0], centroid[1], centroid[2]);
pcl::PointXYZ P2(centroid[0] - coefficient[0] * 10, centroid[1] - coefficient[1] * 10, centroid[2] - coefficient[2] * 10);
viewer->removeAllPointClouds();
viewer->removeAllShapes();
viewer->addPointCloud<pcl::PointXYZ>(BoltCloudFiltered, "cloud");
viewer->setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_COLOR, 1, 1, 1, "cloud");
viewer->setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_POINT_SIZE, 1, "cloud");
viewer->addPointCloud<pcl::PointXYZ>(cloud_plane, "plane");
viewer->setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_COLOR, 0, 1, 0, "plane");
viewer->setPointCloudRenderingProperties(pcl::visualization::PCL_VISUALIZER_POINT_SIZE, 1, "plane");
viewer->addArrow(P2, P1, 1, 0, 0, false);
viewer->addSphere(P1, 0.5, 0, 0, 1);
VecViewer[0]->addArrow(P2, P1, 1, 0, 0, false);
VecViewer[0]->addSphere(P1, 0.5, 0, 0, 1);
}
```
3. **计算所有螺栓的位姿**:
```cpp
void ClassRansac::ComputeAllBolt() {
for (int i = 0; i < BoltNum; ++i) {
ComputeOneBolt(VecBoltPointCloud[i], centroid[i], coefficient[i], VecViewer[i + 1]);
}
}
```
4. **显示结果**:
```cpp
void ClassRansac::DisplayAllPose() {
VecViewer[0]->spinOnce();
}
```
通过上述步骤,代码能够从点云数据中提取螺栓的位置和姿态,并将这些信息显示在可视化窗口中。最终,螺栓在相机坐标系下的坐标可以通过 `centroid` 变量获得。
阅读全文
相关推荐
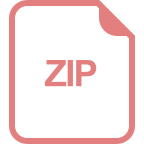
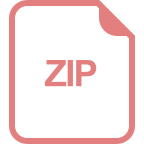


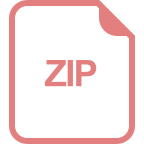












