PYTHON 从数据库表取数据,进行递归操作后插入数据库表
时间: 2024-10-18 19:23:51 浏览: 29
在Python中,使用数据库如SQLAlchemy或pymysql等库,我们可以连接到数据库并执行查询、更新操作。假设你想对一张有递归关联的表(例如,树形结构)进行递归处理,然后将修改后的数据插入回数据库,可以按以下步骤进行:
首先,安装必要的库:
```bash
pip install sqlalchemy pymysql
```
1. 连接数据库:
```python
from sqlalchemy import create_engine, Table, MetaData
# 创建数据库引擎
engine = create_engine('mysql+pymysql://username:password@localhost/dbname')
# 加载元数据
metadata = MetaData()
table_name = 'your_table_name'
your_table = Table(table_name, metadata, autoload_with=engine)
```
2. 设定递归操作函数:
```python
def process_data(parent_id, new_data):
query = your_table.select().where(your_table.c.parent_id == parent_id)
for row in engine.execute(query):
child_data = row.to_dict() # 获取当前行的数据
child_data['new_field'] = new_value # 需要更新的字段
process_data(row.id, child_data) # 递归处理子节点
# 添加新的数据项到表中(这里假设已经完成所有子级处理)
new_row = your_table.insert().values(**new_data).returning(your_table)
new_id = engine.execute(new_row).scalars().one()
# 更新递归关系,链接新添加的节点
update_query = your_table.update().where(
your_table.c.parent_id == parent_id).values(child_id=new_id)
engine.execute(update_query)
# 调用函数,开始递归处理
process_data(starting_parent_id, initial_data)
```
在上述代码中,`starting_parent_id`是你想从哪里开始处理的根节点,`initial_data`则是初始的新数据。
阅读全文
相关推荐
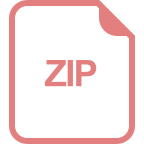
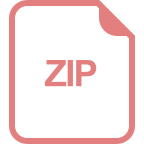
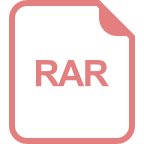
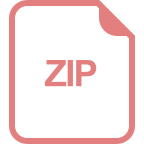
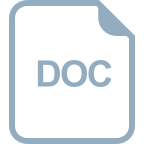
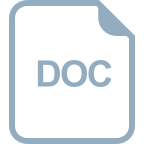
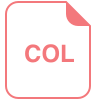
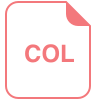
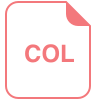
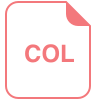
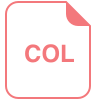
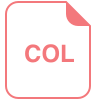
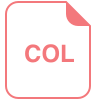
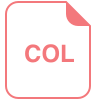
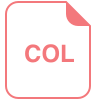
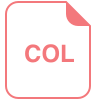
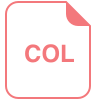
