logistic回归代码matlab
时间: 2023-09-19 13:08:33 浏览: 92
Sure! Here's an example of logistic regression code in MATLAB:
```matlab
% Load the dataset
data = load('dataset.mat');
X = data.X;
y = data.y;
% Feature scaling
X = (X - mean(X)) ./ std(X);
% Add intercept term
X = [ones(size(X, 1), 1), X];
% Initialize parameters
initial_theta = zeros(size(X, 2), 1);
% Set options for fminunc optimization function
options = optimset('GradObj', 'on', 'MaxIter', 400);
% Define the cost function and gradient
costFunction = @(theta) logisticCostFunction(theta, X, y);
[theta, ~] = fminunc(costFunction, initial_theta, options);
% Predict using the learned parameters
probabilities = sigmoid(X * theta);
predictions = probabilities >= 0.5;
% Plot the decision boundary
plotDecisionBoundary(theta, X, y);
% Define the sigmoid function
function g = sigmoid(z)
g = 1 ./ (1 + exp(-z));
end
% Define the cost function and its gradient
function [cost, gradient] = logisticCostFunction(theta, X, y)
m = length(y);
h = sigmoid(X * theta);
cost = (-1 / m) * sum(y .* log(h) + (1 - y) .* log(1 - h));
gradient = (1 / m) * (X' * (h - y));
end
% Define the function to plot the decision boundary
function plotDecisionBoundary(theta, X, y)
plotData(X(:,2:end), y);
hold on;
if size(X, 2) <= 3
plot_x = [min(X(:,2))-2, max(X(:,2))+2];
plot_y = (-1 ./ theta(3)) .* (theta(2) .* plot_x + theta(1));
plot(plot_x, plot_y, '-g', 'LineWidth', 2);
else
u = linspace(-1, 1.5, 50);
v = linspace(-1, 1.5, 50);
z = zeros(length(u), length(v));
for i = 1:length(u)
for j = 1:length(v)
z(i,j) = mapFeature(u(i), v(j)) * theta;
end
end
z = z';
contour(u, v, z, [0, 0], 'LineWidth', 2);
end
hold off;
end
% Define the function to plot the data points
function plotData(X, y)
pos = find(y == 1);
neg = find(y == 0);
scatter(X(pos, 1), X(pos, 2), 'k+', 'LineWidth', 2);
hold on;
scatter(X(neg, 1), X(neg, 2), 'ko', 'MarkerFaceColor', 'y');
hold off;
end
% Define the function to map features for more complex decision boundaries
function out = mapFeature(X1, X2)
degree = 6;
out = ones(size(X1(:,1)));
for i = 1:degree
for j = 0:i
out(:, end + 1) = (X1.^(i-j)) .* (X2.^j);
end
end
end
```
Please make sure to replace `'dataset.mat'` with the actual filename of your dataset.
阅读全文
相关推荐





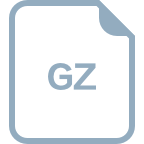












