链表的遍历与删除python代码
时间: 2024-10-23 12:10:40 浏览: 11
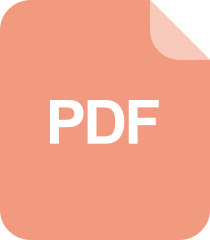
Python 实现链表实例代码
链表的遍历和删除操作在Python中通常是通过节点结构实现的。这里是一个简单的单向链表的示例,包括遍历和删除节点:
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
# 遍历链表
def traverse(self):
current_node = self.head
while current_node is not None:
print(current_node.data, end=" -> ")
current_node = current_node.next
print("None") # 结束打印时显示None
# 删除特定值的节点(如果存在)
def delete_node(self, value):
if self.head is None:
return
# 如果头节点就是目标值
if self.head.data == value:
self.head = self.head.next
return
# 找到前一个节点
previous = self.head
current = self.head.next
while current is not None:
if current.data == value:
previous.next = current.next
return
previous = current
current = current.next
# 使用示例
linked_list = LinkedList()
linked_list.head = Node(1)
second_node = Node(2)
third_node = Node(3)
linked_list.head.next = second_node
second_node.next = third_node
linked_list.traverse() # 输出: 1 -> 2 -> 3 -> None
linked_list.delete_node(2) # 删除值为2的节点
linked_list.traverse() # 输出: 1 -> 3 -> None
```
阅读全文
相关推荐
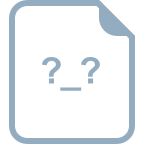
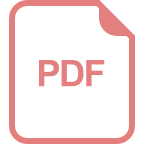


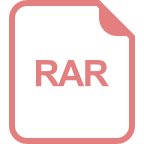
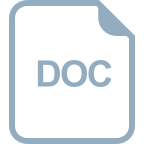
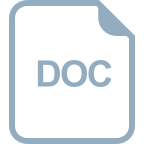
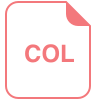










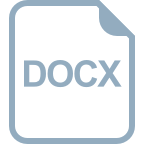