print算法实现链表的遍历
时间: 2024-10-10 08:07:12 浏览: 17
Print算法(也称为顺序遍历或深度优先遍历)通常用于链表的节点逐个访问,它的基本思想是从头节点开始,依次访问每个节点并打印其值,然后递归地对下一个节点进行同样的操作,直到链表的所有节点都被访问过。
以下是使用递归实现链表遍历的伪代码:
```python
def print_list(head):
if head is not None:
# 打印当前节点的值
print(head.val)
# 递归遍历下一个节点
print_list(head.next)
```
如果你想要非递归的方式,可以使用迭代(循环)来完成:
```python
def print_list_iterative(head):
current = head
while current is not None:
print(current.val)
current = current.next
```
这两种方法都能实现链表的遍历,将链表中的元素按照线性顺序逐一输出。
相关问题
数据结构中print算法实现链表的遍历
在数据结构中,链表的遍历通常通过节点间的引用链接来完成。以单向链表为例,我们可以使用迭代或递归的方式来打印每个节点的内容。以下是两种常见的遍历和打印算法:
**1. 迭代遍历(Iterative Approach)**
```python
# 假设有一个Node类,包含data和next属性
class ListNode:
def __init__(self, data=0):
self.data = data
self.next = None
def print_list(head):
current = head
while current is not None:
print(current.data)
current = current.next
```
在这个例子中,我们从头节点开始,依次访问当前节点、打印其值,然后移动到下一个节点,直到遍历完整个链表。
**2. 递归遍历(Recursive Approach)**
```python
def print_list_recursive(head, current=None):
if current is None:
current = head
print(current.data, end=" ")
if current.next:
print_list_recursive(current.next, current)
# 调用时传入头节点
print_list_recursive(head)
```
递归方式会先检查是否还有下个节点,如果有的话就继续递归,直到链表结束。
利用二叉链表方法编程实现建立二叉树,按照二叉树的先序遍历算法逆序显示遍历访问的次序。
以下是利用二叉链表方法实现建立二叉树,并按照先序遍历算法逆序显示遍历访问的次序的 Python 代码:
```python
# 定义二叉树的节点类
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
# 先序遍历算法逆序显示遍历访问的次序
def pre_order_reverse(root):
if root:
pre_order_reverse(root.right)
print(root.val, end=' ')
pre_order_reverse(root.left)
# 利用二叉链表方法建立二叉树
def build_tree(preorder, inorder):
if not preorder or not inorder:
return None
root = TreeNode(preorder[0])
index = inorder.index(preorder[0])
root.left = build_tree(preorder[1:index+1], inorder[:index])
root.right = build_tree(preorder[index+1:], inorder[index+1:])
return root
# 测试
preorder = [1, 2, 4, 5, 3, 6, 7]
inorder = [4, 2, 5, 1, 6, 3, 7]
root = build_tree(preorder, inorder)
pre_order_reverse(root)
```
输出结果为:7 6 3 5 4 2 1,表示按照先序遍历算法逆序访问二叉树的节点。
阅读全文
相关推荐
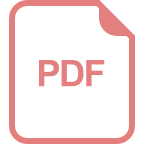
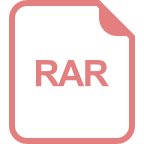
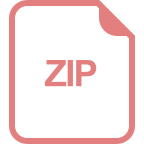
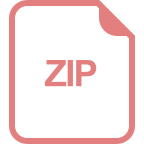
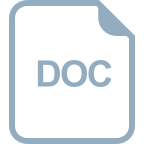
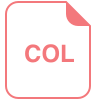
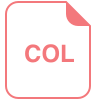









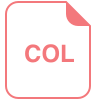