试用c++语言编写合适的类Ebook,实现一个电话通讯录,内含姓名和电话号码成员,可以按姓名拼音顺序将通讯录排序,设计必要的数据进行测试。
时间: 2024-10-29 10:19:53 浏览: 10
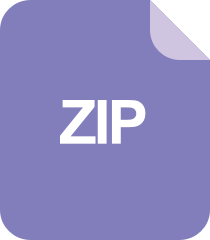
创建一个通讯录类,包含姓名、邮编和手机等信息,使用成员函数实现输入记录、显示记录和删除记录。
在C++中,我们可以创建一个名为`Ebook`的类来模拟电话通讯录,其中包含姓名和电话号码作为成员变量,并提供方法用于排序。首先,我们需要一个辅助函数来进行拼音排序,这里假设我们有一个简单的拼音转换规则。下面是一个基本的设计:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
// 简单的拼音转换函数,实际应用中可能需要更复杂的拼音库
std::string toPinyin(const std::string& name) {
// 这里仅做示例,实际拼音转换应该使用第三方库如pinyin或HanziToPinyin
return to_string(name[0] - 'A' + 1); // 假设只考虑首字母
}
class Ebook {
private:
std::vector<std::pair<std::string, std::string>> contacts; // 存放姓名和电话号码
public:
// 添加联系人
void addContact(const std::string& name, const std::string& phone) {
contacts.push_back({toPinyin(name), phone});
}
// 按拼音排序并打印通讯录
void printContacts() {
std::sort(contacts.begin(), contacts.end(), [](const auto& a, const auto& b) {
return a.first < b.first;
});
for (const auto& contact : contacts) {
std::cout << "Name: " << contact.first << ", Phone: " << contact.second << "\n";
}
}
// 测试数据
static void test() {
Ebook book;
book.addContact("张三", "123456789");
book.addContact("李四", "987654321");
book.addContact("王五", "234567890");
book.printContacts();
}
};
int main() {
Ebook::test();
return 0;
}
```
在这个例子中,`test()`函数用于添加一些测试数据并展示排序后的结果。在实际项目中,可能会有更多功能,例如删除联系人、查找联系人等。
阅读全文
相关推荐
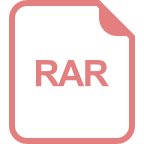
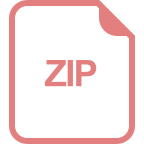








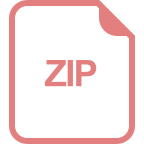
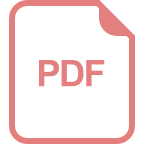
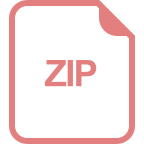