c++试编写合适的类Ebook,实现一个电话通讯录,内含姓名和电话号码成员,可以按姓名拼音顺序将通讯录排序
时间: 2024-10-29 14:09:01 浏览: 8
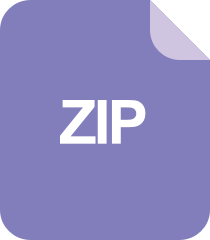
创建一个通讯录类,包含姓名、邮编和手机等信息,使用成员函数实现输入记录、显示记录和删除记录。
在C++中,我们可以创建一个名为`Ebook`的类,用于模拟电话通讯录的功能,其中包含姓名(`Name`)和电话号码(`PhoneNumber`)两个基本属性,并提供一种方法来进行按姓名拼音排序。这里我们假设有一个简单的拼音转换函数`toPinyin`来进行拼音化处理。以下是类`Ebook`的一个简单实现:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
// 模拟拼音转换,实际应用中可能需要外部库支持
std::string toPinyin(const std::string& name) {
// 这里仅作示例,实际拼音转换更复杂,需要借助拼音库或API
return "pinyin_of_" + name;
}
class Ebook {
private:
std::vector<std::pair<std::string, std::string>> contacts; // 存储姓名和电话
public:
void addContact(const std::string& name, const std::string& phoneNumber) {
contacts.push_back({toPinyin(name), phoneNumber});
}
void sortContacts() {
std::sort(contacts.begin(), contacts.end(), [](const auto& a, const auto& b) {
return a.first < b.first; // 按拼音升序排列
});
}
void printContacts() const {
for (const auto& contact : contacts) {
std::cout << "姓名: " << contact.first << ", 电话: " << contact.second << '\n';
}
}
};
int main() {
Ebook myEbook;
// 添加联系人
myEbook.addContact("张三", "123456");
myEbook.addContact("李四", "789012");
myEbook.addContact("王五", "345678");
// 排序联系人
myEbook.sortContacts();
// 打印通讯录
myEbook.printContacts();
return 0;
}
```
阅读全文
相关推荐
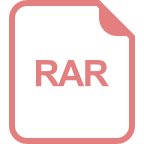
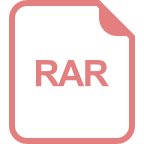







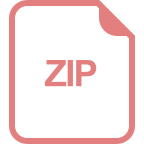
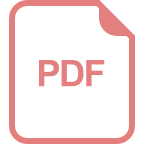
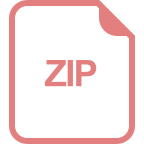