使用c++函数编写合适的类Ebook,实现一个电话通讯录,内含姓名和电话号码成员,可以按姓名拼音顺序将通讯录排序,设计必要的数据进行测试。
时间: 2024-10-29 21:13:00 浏览: 42
在C++中,我们可以创建一个名为`Ebook`的类来模拟电话通讯录的功能。首先,我们需要定义一个基础的电话联系人`Contact`类,包含姓名`name`和电话号码`phoneNumber`作为成员变量。然后,我们可以在`Ebook`类中实现排序功能,并提供添加、查找和删除联系人的方法。
```cpp
#include <iostream>
#include <string>
#include <map>
#include <algorithm>
// 基础联系人类
class Contact {
public:
std::string name;
std::string phoneNumber;
// 构造函数
Contact(const std::string& n, const std::string& p) : name(n), phoneNumber(p) {}
};
// 按拼音排序的辅助结构
struct CompareByName {
bool operator()(const Contact& c1, const Contact& c2) {
return comparePinyin(c1.name, c2.name) < 0; // 假设comparePinyin是一个能比较姓名拼音的函数
}
};
// 电话通讯录类
class Ebook {
private:
std::map<std::string, Contact, CompareByName> contactList;
public:
// 添加联系人
void addContact(const std::string& name, const std::string& phoneNumber) {
if (contactList.find(name) == contactList.end()) {
contactList[name] = Contact{name, phoneNumber};
std::sort(contactList.begin(), contactList.end());
} else {
std::cout << "Contact with the same name already exists!" << std::endl;
}
}
// 查找联系人
Contact findContact(const std::string& name) const {
auto it = contactList.find(name);
if (it != contactList.end())
return it->second;
else
throw std::runtime_error("Contact not found.");
}
// 删除联系人
void removeContact(const std::string& name) {
if (contactList.erase(name)) {
std::sort(contactList.begin(), contactList.end());
} else {
std::cout << "Contact not found to delete." << std::endl;
}
}
// 打印全部联系人列表
void printContacts() const {
for (const auto& entry : contactList) {
std::cout << "Name: " << entry.first << ", Phone: " << entry.second.phoneNumber << std::endl;
}
}
};
int main() {
Ebook phoneBook;
phoneBook.addContact("张三", "12345678");
phoneBook.addContact("李四", "98765432");
phoneBook.printContacts();
phoneBook.removeContact("张三");
phoneBook.printContacts();
try {
phoneBook.findContact("未知"); // 这里假设comparePinyin的返回值用于判断是否找到对应的联系人
} catch (const std::exception& e) {
std::cout << "Error: " << e.what() << std::endl;
}
return 0;
}
```
在这个例子中,我们通过`CompareByName`结构体实现了按姓名拼音排序。注意,这里假定了一个`comparePinyin`函数用于比较姓名的拼音,实际应用中需要实现这个函数或使用现成的拼音库。
阅读全文
相关推荐
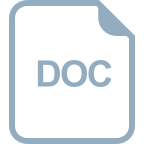
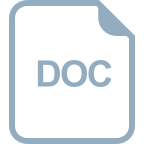
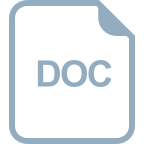





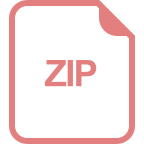


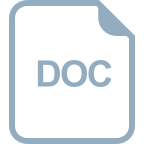
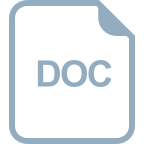
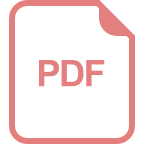
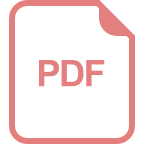
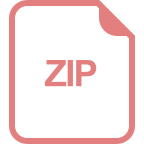