用类java学生信息管理系统
时间: 2024-12-27 09:24:35 浏览: 3
### 创建基于Java的学生信息管理系统
#### 设计思路
为了构建一个简单而高效的学生信息系统,可以采用面向对象编程(OOP)的原则来设计类结构。主要涉及几个核心实体:`Student`, `Course` 和 `SystemManager`. 这些实体之间的关系可以通过关联、聚合或组合的方式建立起来[^1].
#### 类图概述
- **Student**: 存储学生的个人信息以及所选课程列表.
- **Course**: 表示一门具体的学科, 包含名称、编号等属性.
- **SystemManager**: 提供增删改查等功能接口.
#### 实现细节
##### Student.java 文件定义如下:
```java
public class Student {
private String id;
private String name;
private List<Course> courses;
public Student(String id, String name){
this.id = id;
this.name = name;
this.courses = new ArrayList<>();
}
// Getters and Setters...
}
```
##### Course.java 定义如下:
```java
public class Course{
private final String courseId;
private final String courseName;
public Course(String courseId, String courseName){
this.courseId = courseId;
this.courseName = courseName;
}
// Getters ...
}
```
##### SystemManager.java 中实现了基本操作功能:
```java
import java.util.*;
public class SystemManager {
Map<String, Student> studentMap = new HashMap<>();
/**
* 添加新学生记录到系统中
*/
void addNewStudent(Student s){
if (!studentMap.containsKey(s.getId())){
studentMap.put(s.getId(),s);
}else{
throw new RuntimeException("该学号已存在!");
}
}
/**
* 删除指定ID的学生数据
*/
boolean removeStudentById(String id){
return studentMap.remove(id)!=null;
}
/**
* 更新现有学生的信息
*/
void updateStudentInfo(Student updatedS){
if (studentMap.containsKey(updatedS.getId())) {
studentMap.replace(updatedS.getId(),updatedS);
} else {
throw new NoSuchElementException();
}
}
/**
* 查询特定条件下的所有匹配项
*/
Collection<Student> queryStudents(Predicate<Student> predicate){
return studentMap.values().stream()
.filter(predicate)
.collect(Collectors.toList());
}
}
```
上述代码片段展示了如何利用集合框架中的`HashMap`存储键值对学生对象,并通过流式API简化查询逻辑[^2].
阅读全文
相关推荐
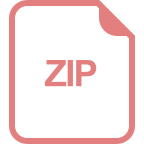
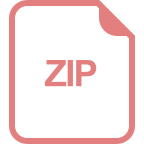
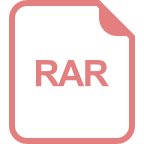
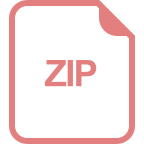
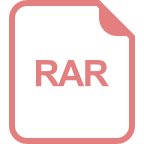
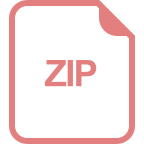
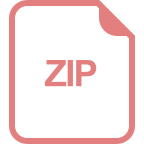
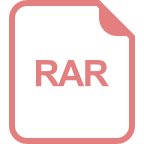
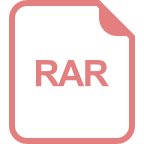
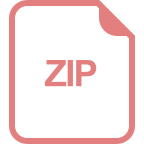
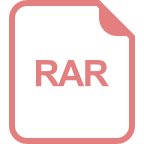
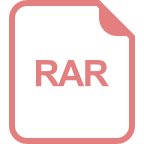
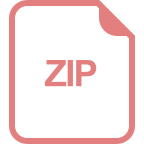
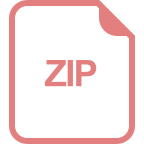
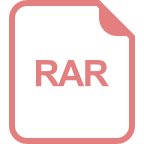
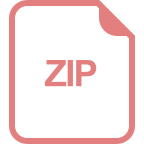
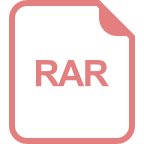