cv2.HoughLinesP()
时间: 2023-10-19 09:57:32 浏览: 47
cv2.HoughLinesP()是OpenCV中的一个函数,用于检测图像中的直线。它基于Hough变换,可以找到图像中的直线段。
这个函数的使用方法如下:
```
lines = cv2.HoughLinesP(image, rho, theta, threshold, minLineLength, maxLineGap)
```
其中,参数的含义如下:
- `image`:输入图像,通常是灰度图像。
- `rho`:以像素为单位的距离精度,一般设置为1。
- `theta`:以弧度为单位的角度精度,一般设置为np.pi/180。
- `threshold`:直线投票阈值,只有得票数超过这个阈值的直线才会被保留。
- `minLineLength`:最小直线长度,比这个阈值短的直线会被丢弃。
- `maxLineGap`:最大直线间隙,如果两条直线之间的间隙小于这个阈值,则认为它们是同一条直线。
函数会返回一个包含检测到的直线的数组lines,每条直线由两个端点的坐标表示。
需要注意的是,cv2.HoughLinesP()只能检测出直线的端点,如果需要得到完整的直线,可以使用cv2.line()函数来绘制。
相关问题
cv2.HoughLinesP
cv2.HoughLinesP函数是OpenCV中的一种霍夫变换算法的实现,用于检测图像中直线的位置。霍夫变换是一种常用的图像处理技术,可以将图像中的直线、圆等图形检测出来。cv2.HoughLinesP函数在霍夫变换的基础上,可以直接输出直线的端点坐标,便于后续的进一步处理。其函数原型为:
```python
lines = cv2.HoughLinesP(image, rho, theta, threshold, minLineLength, maxLineGap)
```
其中,参数解释如下:
- image:输入图像,必须是单通道二进制图像,即只有黑白两种颜色。
- rho:表示直线距离原点的最小单位,一般取值为 1。
- theta:表示直线角度的最小单位,一般取值为 np.pi/180。
- threshold:表示检测直线的阈值,只有检测到的直线的端点数目大于该值才被认为是一条直线。
- minLineLength:表示检测出的直线的最小长度,小于该长度的直线将被忽略。
- maxLineGap:表示检测出的直线的最大间隔,小于该间隔的直线将被视为一条直线。
返回值 lines 是一个 numpy 数组,每一行表示检测到的一条直线,其中包含四个元素,表示直线的两个端点的坐标。
cv2.houghlines和cv2.HoughLinesP、
`cv2.HoughLines` 和 `cv2.HoughLinesP` 都是 OpenCV 中用于直线检测的函数,但它们在参数和功能上有所不同。
`cv2.HoughLines` 函数使用标准的霍夫变换算法来检测直线。它返回的是检测到的直线的参数 rho 和 theta。需要注意的是,`cv2.HoughLines` 函数只能检测出直线的参数,需要进一步处理才能绘制出直线或者获取直线的端点坐标。
`cv2.HoughLinesP` 函数使用改进的霍夫变换算法来检测直线,称为随机霍夫变换。相比于 `cv2.HoughLines`,`cv2.HoughLinesP` 返回的是直线的端点坐标,而不仅仅是直线的参数 rho 和 theta。这样可以更方便地绘制直线或者进行后续处理。
函数调用的语法如下:
```python
lines = cv2.HoughLinesP(image, rho, theta, threshold, minLineLength, maxLineGap)
```
- `image`:输入的二值化图像,通常是通过 Canny 边缘检测等方法得到的。
- `rho`:以像素为单位的距离精度。一般设为 1。
- `theta`:以弧度为单位的角度精度。一般设为 np.pi/180。
- `threshold`:阈值参数,用于确定检测到直线的最小投票数。
- `minLineLength`:最小直线长度,比这个长度短的直线将被丢弃。
- `maxLineGap`:最大直线间隙,如果两条直线之间的间隙大于这个值,则被认为是两条不同的直线。
需要注意的是,`cv2.HoughLinesP` 函数返回的直线是以端点坐标 (x1, y1, x2, y2) 表示的。
相关推荐
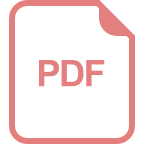












