给出详细的函数例子,适用于五种正交多项本的最小二乘法拟合
时间: 2024-10-17 20:14:05 浏览: 34
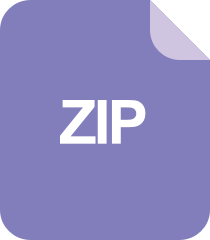
zjqxszx.zip_matlab散点拟合_散点拟合_最小二乘法拟合_正交多项式_点拟合
五种常见的正交多项式包括线性、多项式、Legendre、Hermite和Chebyshev。这里我会给出一个简单的例子来展示如何分别使用这些正交多项式进行最小二乘法拟合。
### 1. 线性多项式 (Least Squares Linear Regression)
```python
import numpy as np
# 假设我们有一个数据点列表 (x, y)
x = np.array([1, 2, 3, 4, 5])
y = np.array([2, 4, 5, 4, 5])
# 线性模型:y = a * x + b
A = np.vstack([np.ones(len(x)), x]).T
a, b = np.linalg.inv(A.T @ A) @ A.T @ y
print(f"a = {a}, b = {b}")
# 线性最小二乘拟合的函数 yhat = a * x + b
def linear_fit(x):
return a * x + b
```
### 2. 多项式拟合 (Polynomial Regression)
```python
from sklearn.preprocessing import PolynomialFeatures
# 高阶多项式,例如二次
degree = 2
poly = PolynomialFeatures(degree=degree)
X_poly = poly.fit_transform(x[:, np.newaxis])
# 最小二乘拟合
model = LinearRegression()
model.fit(X_poly, y)
coeffs = model.coef_
print(f"Polyfit coefficients: {coeffs}")
def poly_fit(x):
return np.dot(poly.transform(x), coeffs)
```
### 3. Legendre多项式 (Legendre Polynomials)
```python
from scipy.special import legendre
# 初始化Legendre多项式
basis = legendre(degree)
# 拟合函数
def legendre_fit(x):
return basis.dot(coeffs) # 假设coeffs存储的是Legendre多项式的系数
```
其他两种,Hermite和Chebyshev,可以用类似的方法,但可能涉及到特定库如`scipy.special`或者`numpy.polynomial`来进行计算。对于这两种,系数的计算可能会稍微复杂一些,需要了解相应的递推公式或函数。
阅读全文
相关推荐
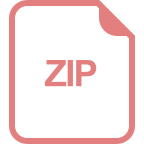
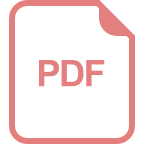
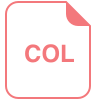
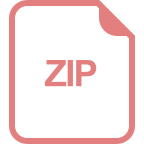
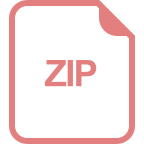
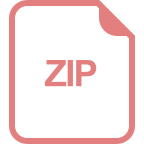