C#,窗体Form1创建了UDP的接收端和发送端,当Form1接收到数据“anniu1”时,Form2窗体上的panel的背景图片换成其它图片
时间: 2024-09-11 13:08:57 浏览: 74
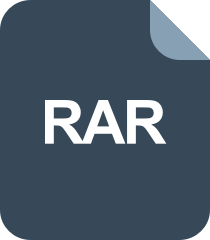
js-anniu.rar_js切换页面
在C#中,为了实现这样的功能,你需要做以下几个步骤:
1. **在Form1上设置UDP接收**:
- 创建一个`UdpClient`实例,用于接收UDP数据。
- 使用`ReceiveAsync()`方法异步接收数据,并在完成事件中处理接收到的数据。
```csharp
private void Form1_Load(object sender, EventArgs e)
{
UdpClient udpClient = new UdpClient(YourPortNumber);
IPEndPoint remoteEndPoint = new IPEndPoint(IPAddress.Any, YourListenPort);
udpClient.BeginReceive(new AsyncCallback(ReceiveData), null, remoteEndPoint);
}
private async void ReceiveData(IAsyncResult ar)
{
UdpClient udpClient = (UdpClient)ar.AsyncState;
byte[] data = await udpClient.EndReceive(ar, ref remoteEndPoint);
string receivedMessage = Encoding.ASCII.GetString(data);
if (receivedMessage == "anniu1")
{
// 发送到Form2
Invoke(new Action(() => ShowNewImageOnForm2()));
}
}
```
2. **在Form1中触发显示新图像的事件**:
- `Invoke()`是为了保证UI更新在主线程中安全进行,避免可能出现的跨线程异常。
- `ShowNewImageOnForm2`方法需要传递给Form2以更改其Panel的背景图片。
```csharp
private void ShowNewImageOnForm2()
{
if (Form2 != null && Form2.Visible)
{
Form2 panleControl = Form2.FindForm().FindChild<Form2>();
if (panleControl != null)
{
// 更换图片逻辑
panleControl.BackgroundImage = YourNewImage;
}
}
}
```
3. **在Form2中接收并处理来自Form1的消息**:
- 可能需要在Form2中添加一个公开的委托或者事件处理来监听Form1传递过来的图像切换信号。
请注意替换上述代码中的`YourPortNumber`, `YourListenPort`, 和 `YourNewImage` 为实际的值或变量。
阅读全文
相关推荐
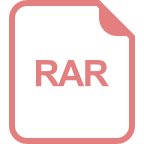
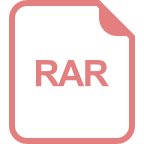




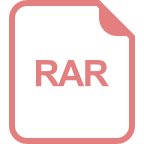
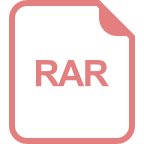









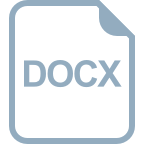