用c++写一个程序:输入一个不超过80个字符的字符串,分别统计其中英文大写字母、数字、空格的字符的个数
时间: 2024-12-13 09:19:18 浏览: 22
在C++中,可以使用标准库中的`ctype`函数配合`std::cin`读取用户输入,并使用计数器逐个统计英文大写字母、数字和空格的数量。以下是完整的代码示例:
```cpp
#include <iostream>
#include <cctype> // 包含字符分类函数
using namespace std;
int countLetters(string str);
int countDigits(string str);
int countSpaces(string str);
int main() {
string input;
cout << "请输入一个不超过80个字符的字符串:";
getline(cin, input); // 使用getline获取包含空格的完整行
if (input.length() > 80) {
cout << "输入的字符串超过了80个字符,请重新输入。\n";
return 0;
}
int upperCase = countLetters(input);
int digits = countDigits(input);
int spaces = countSpaces(input);
cout << "英文大写字母数量:" << upperCase << "\n";
cout << "数字数量:" << digits << "\n";
cout << "空格数量:" << spaces << "\n";
return 0;
}
// 统计大写字母的函数
int countLetters(string str) {
int count = 0;
for (char c : str) {
if (isupper(c)) { // 检查是否为大写字母
count++;
}
}
return count;
}
// 统计数字的函数
int countDigits(string str) {
int count = 0;
for (char c : str) {
if (isdigit(c)) { // 检查是否为数字
count++;
}
}
return count;
}
// 统计空格的函数
int countSpaces(string str) {
int count = count whitespaces in str; // 空白符包括空格、制表符、换页符等
return count;
}
```
请注意,上述代码中的`count whitespaces in str`并没有直接给出,因为C++标准库并没有提供直接计算字符串中空白字符数量的函数。如果你需要处理所有空白符(如空格、制表符等),你需要自定义一个函数或者利用第三方库来实现。
阅读全文
相关推荐
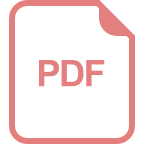
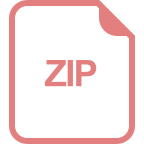
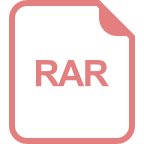















