python实现利用中点算法绘制两条直线代码
时间: 2024-10-17 10:05:18 浏览: 37
在Python中,你可以使用matplotlib库来绘制图形,其中中点算法(也称为线段连接法)通常用于创建折线图。这里是一个简单的例子,演示如何通过连接两个点来绘制一条直线:
```python
import matplotlib.pyplot as plt
# 定义两点坐标
point1 = (0, 0)
point2 = (5, 4) # 这里可以替换为你想要连接的任意两点
# 计算并存储中间点的x和y坐标
mid_x = (point1[0] + point2[0]) / 2
mid_y = (point1[1] + point2[1]) / 2
# 创建一个新的数据点列表
points = [point1, mid_point, point2]
# 创建一个新的图表
plt.figure()
# 绘制线段
plt.plot(points)
# 显示图表
plt.show()
```
在这个示例中,`mid_point`代表了连接`point1`和`point2`的中点。如果你想连续绘制多条直线,只需添加更多的点。
如果你需要更复杂的线性拟合或者其他类型的绘图操作,中点算法可能会更适合简单直线的绘制,对于复杂的曲线可能需要其他方法如贝塞尔曲线等。
相关问题
python实现利用中点算法绘制两条直线代码(1)分别是:L1(Point(20, 10), Point(100, 180)), L2(Point(-50, 100), Point(100, -50))。
当然,我们可以按照上面提供的示例稍作调整来绘制这两条线及其中点连接线。这里直接给出代码:
```python
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.patches import Circle # 为了添加点标记
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
# 定义两条线的起点和终点
L1 = Point(20, 10) and Point(100, 180)
L2 = Point(-50, 100) and Point(100, -50)
# 计算中点
midpoint_L1 = ((L1[0].x + L1[1].x) / 2, (L1[0].y + L1[1].y) / 2)
midpoint_L2 = ((L2[0].x + L2[1].x) / 2, (L2[0].y + L2[1].y) / 2)
# 创建绘图环境
plt.figure(figsize=(8, 6))
# 绘制两条线
plt.plot([L1[0].x, L1[1].x], [L1[0].y, L1[1].y], 'r-', label=f'Line 1: {L1}')
plt.plot([L2[0].x, L2[1].x], [L2[0].y, L2[1].y], 'b-', label=f'Line 2: {L2}')
# 在点上添加标记(圆圈)
circle1 = Circle((L1[0].x, L1[0].y), 2, color='r', fill=True)
circle2 = Circle((L1[1].x, L1[1].y), 2, color='r', fill=True)
circle3 = Circle((L2[0].x, L2[0].y), 2, color='b', fill=True)
circle4 = Circle((L2[1].x, L2[1].y), 2, color='b', fill=True)
plt.gca().add_patch(circle1)
plt.gca().add_patch(circle2)
plt.gca().add_patch(circle3)
plt.gca().add_patch(circle4)
# 绘制中点连线
plt.plot([L1[0].x, midpoint_L1[0]], [L1[0].y, midpoint_L1[1]], 'g--')
plt.plot([midpoint_L1[0], midpoint_L2[0]], [midpoint_L1[1], midpoint_L2[1]], 'c--')
# 添加标签
plt.title('Drawing Lines with Midpoints')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
# 显示图形
plt.grid(True)
plt.show()
```
在这个版本中,我还增加了对每个端点的小圆圈标记,并设置了网格线。注意,`Point` 类在这里只是一个简化的表示,如果需要的话可以扩展它的功能。
python利用中点算法绘制两条直线,分别是:L1(Point(20, 10), Point(100, 180)), L2(Point(-50, 100), Point(100, -50))。
Python中可以使用matplotlib库来绘制二维图形,其中中点算法通常用于连接两点形成线段,而这里是直接给出两点坐标来绘制直线。首先,你需要安装matplotlib,如果你还没有安装,可以使用pip install matplotlib命令。
以下是绘制两条直线L1和L2的简单示例:
```python
import matplotlib.pyplot as plt
import numpy as np
# 定义直线的两个端点
point1_L1 = (20, 10)
point2_L1 = (100, 180)
point1_L2 = (-50, 100)
point2_L2 = (100, -50)
# 绘制L1直线
plt.plot([point1_L1[0], point2_L1[0]], [point1_L1[1], point2_L1[1]])
# 绘制L2直线
plt.plot([point1_L2[0], point2_L2[0]], [point1_L2[1], point2_L2[1]])
# 添加标题和标签
plt.title('Two Lines in Python')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
# 显示图像
plt.show()
```
运行这段代码后,你会看到一条从(20, 10)到(100, 180)的直线和另一条从(-50, 100)到(100, -50)的直线。
阅读全文
相关推荐
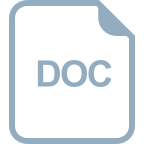
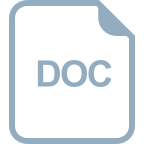
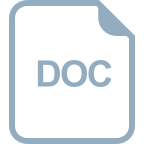







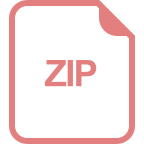





