#include <QGraphicsLineItem>
时间: 2024-10-01 07:10:55 浏览: 32
`#include <QGraphicsLineItem>` 是 C++ 中使用的预处理器指令,它引入了 Qt Graphics 模块下的 QGraphicsLineItem 类。QGraphicsLineItem 是一个 QGraphicsItem 的子类,专门用于在 QGraphicsView 或 QGraphicsScene 中绘制二维线条。它可以独立存在,也可以作为其他图形元素(如 QGraphicsRectItem、QGraphicsEllipseItem 等)的一部分。
当你需要在 Qt 应用程序中创建和操控像直线、虚线这样的几何形状时,可以使用 QGraphicsLineItem。这个类提供了设置起点、终点、颜色、宽度等属性的方法,以及一些用于移动、调整方向等的操作函数。
比如,你可以创建一个 QGraphicsLineItem,并将其添加到场景中:
```cpp
QGraphicsLineItem *line = new QGraphicsLineItem(QLineF(startX, startY, endX, endY), scene);
scene.addItem(line); // 将线添加到指定的场景中
```
这里,`QLineF` 定义了线段的起点和终点。
相关问题
在qt中使用Qvector<QPointf>记录路径节点,连接图元,鼠标移动线段,线段随着变动例子
好的,下面是一个简单的例子,演示如何在Qt中使用QVector<QPointF>记录路径节点,连接图元,鼠标移动线段,线段随着变动。
MainWindow.h文件:
```c++
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include <QPainter>
#include <QMouseEvent>
#include <QGraphicsScene>
#include <QGraphicsView>
#include <QGraphicsLineItem>
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
protected:
void paintEvent(QPaintEvent *event);
void mousePressEvent(QMouseEvent *event);
void mouseMoveEvent(QMouseEvent *event);
void mouseReleaseEvent(QMouseEvent *event);
private:
Ui::MainWindow *ui;
QVector<QPointF> m_points;
bool m_isDrawing;
QGraphicsScene* m_scene;
QGraphicsView* m_view;
QGraphicsLineItem* m_line;
};
#endif // MAINWINDOW_H
```
MainWindow.cpp文件:
```c++
#include "mainwindow.h"
#include "ui_mainwindow.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
// 创建场景和视图
m_scene = new QGraphicsScene(this);
m_view = new QGraphicsView(m_scene, this);
m_view->setGeometry(10, 50, 780, 500);
m_view->setRenderHint(QPainter::Antialiasing);
// 初始化
m_isDrawing = false;
m_line = nullptr;
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::paintEvent(QPaintEvent *event)
{
Q_UNUSED(event);
QPainter painter(this);
painter.setRenderHint(QPainter::Antialiasing);
painter.setPen(QPen(Qt::red, 2));
// 绘制路径节点
for (int i = 0; i < m_points.size(); ++i)
{
painter.drawEllipse(m_points[i], 3, 3);
}
// 绘制线段
for (int i = 0; i < m_points.size() - 1; ++i)
{
painter.drawLine(m_points[i], m_points[i + 1]);
}
}
void MainWindow::mousePressEvent(QMouseEvent *event)
{
if (event->button() == Qt::LeftButton)
{
// 添加节点
m_points.append(event->pos());
m_isDrawing = true;
// 创建线段item
m_line = new QGraphicsLineItem(QLineF(event->pos(), event->pos()));
m_line->setPen(QPen(Qt::red, 2));
m_scene->addItem(m_line);
}
}
void MainWindow::mouseMoveEvent(QMouseEvent *event)
{
if (m_isDrawing)
{
// 更新节点
m_points.last() = event->pos();
// 更新线段item
m_line->setLine(QLineF(m_points[m_points.size() - 2], event->pos()));
// 刷新窗口
update();
}
}
void MainWindow::mouseReleaseEvent(QMouseEvent *event)
{
if (event->button() == Qt::LeftButton)
{
// 添加节点
m_points.last() = event->pos();
m_isDrawing = false;
// 更新线段item
m_line->setLine(QLineF(m_points[m_points.size() - 2], event->pos()));
// 刷新窗口
update();
}
}
```
在这个例子中,我们使用QPainter在窗口中绘制路径节点和线段,并使用QGraphicsView和QGraphicsScene来实现鼠标移动线段和线段随着变动的效果。
希望这个例子能够帮助您理解如何在Qt中使用QVector<QPointF>记录路径节点,连接图元,鼠标移动线段,线段随着变动。
QGraphicsLineItem C++ code
Here is an example of how to create a `QGraphicsLineItem` in C++:
```
#include <QGraphicsScene>
#include <QGraphicsView>
#include <QGraphicsLineItem>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QGraphicsScene scene;
QGraphicsView view(&scene);
// Create a line item with starting point (0, 0) and ending point (100, 100)
QGraphicsLineItem *line = new QGraphicsLineItem(0, 0, 100, 100);
line->setPen(QPen(Qt::red, 2)); // Set color and width of line
scene.addItem(line);
view.show();
return app.exec();
}
```
This code creates a `QGraphicsScene` and a `QGraphicsView` to display the scene. It then creates a `QGraphicsLineItem` with starting point (0, 0) and ending point (100, 100), sets the color and width of the line using a `QPen`, adds the line to the scene using `addItem()`, and shows the view.
阅读全文
相关推荐
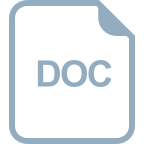
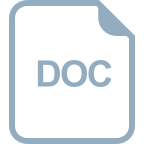
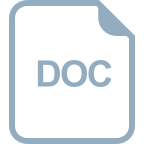


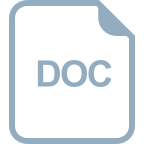
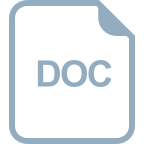
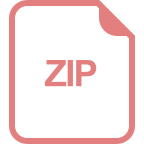
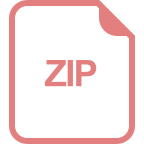
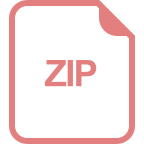
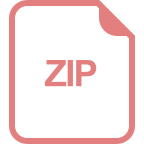
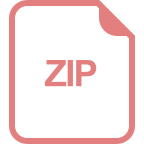
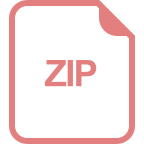