在qt中使用c++,写一个类,继承QGraphicsPathItem,QGraphicsLineItem,实现用鼠标点击选择连接折线方式
时间: 2024-02-16 12:02:48 浏览: 34
好的,我可以帮你解决这个问题。
首先,你需要在类的头文件中声明继承自QGraphicsPathItem和QGraphicsLineItem:
```c++
#include <QGraphicsPathItem>
#include <QGraphicsLineItem>
class MyItem : public QGraphicsPathItem, public QGraphicsLineItem
{
Q_OBJECT
```
接下来,你需要在类的构造函数中创建连接折线的路径和线段:
```c++
public:
MyItem(QGraphicsItem *parent = nullptr) : QGraphicsPathItem(parent), QGraphicsLineItem(parent)
{
// 设置连接折线的路径
QPainterPath path;
path.moveTo(0, 0);
path.lineTo(50, 0);
path.lineTo(50, 50);
setPath(path);
// 设置连接折线的线段
QLineF line(50, 50, 100, 100);
setLine(line);
}
```
然后,你需要在类中实现鼠标点击事件的处理函数:
```c++
protected:
void mousePressEvent(QGraphicsSceneMouseEvent *event) override
{
// 如果鼠标点击在连接折线上,则选中该折线
if (path().contains(event->pos()) || line().contains(event->pos()))
{
setSelected(true);
}
else
{
QGraphicsPathItem::mousePressEvent(event);
QGraphicsLineItem::mousePressEvent(event);
}
}
```
最后,别忘了在类的头文件中添加Q_OBJECT宏和声明mousePressEvent函数。
完整代码如下:
```c++
#include <QGraphicsPathItem>
#include <QGraphicsLineItem>
class MyItem : public QGraphicsPathItem, public QGraphicsLineItem
{
Q_OBJECT
public:
MyItem(QGraphicsItem *parent = nullptr) : QGraphicsPathItem(parent), QGraphicsLineItem(parent)
{
// 设置连接折线的路径
QPainterPath path;
path.moveTo(0, 0);
path.lineTo(50, 0);
path.lineTo(50, 50);
setPath(path);
// 设置连接折线的线段
QLineF line(50, 50, 100, 100);
setLine(line);
}
protected:
void mousePressEvent(QGraphicsSceneMouseEvent *event) override
{
// 如果鼠标点击在连接折线上,则选中该折线
if (path().contains(event->pos()) || line().contains(event->pos()))
{
setSelected(true);
}
else
{
QGraphicsPathItem::mousePressEvent(event);
QGraphicsLineItem::mousePressEvent(event);
}
}
};
```
希望这能帮助到你!
相关推荐
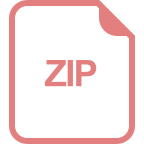














