C++Qt实现multisim中复制拖拽器件并且器件之间连接起来的功能
时间: 2023-12-30 13:07:43 浏览: 72
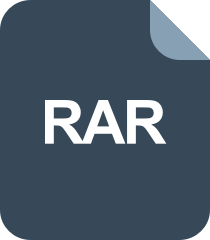
基于c++的qt多线程
实现multisim中复制拖拽器件并且器件之间连接起来的功能可以分为以下几个步骤:
1. 实现器件的拖拽:使用Qt的QGraphicsView和QGraphicsItem实现器件的拖拽功能。可以通过重载QGraphicsItem的mousePressEvent、mouseMoveEvent和mouseReleaseEvent函数来实现对鼠标事件的响应,从而实现拖拽功能。
2. 实现器件的复制:可以在鼠标释放事件中判断是否需要进行复制操作,如果需要,则可以通过创建一个新的QGraphicsItem对象,并将其添加到场景中来实现复制功能。
3. 实现器件之间的连接:可以通过在鼠标释放事件中判断当前鼠标位置是否在另一个器件的连接点上,如果是,则可以创建一个新的连接线QGraphicsLineItem,并将其添加到场景中来实现连接功能。
下面是一个简单的示例代码,演示如何实现这些功能:
```c++
#include <QtWidgets>
class CircuitItem : public QGraphicsRectItem
{
public:
CircuitItem(QGraphicsItem *parent = nullptr)
: QGraphicsRectItem(parent)
{
setRect(0, 0, 100, 50);
setFlag(QGraphicsItem::ItemIsMovable, true);
}
void paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget) override
{
// 绘制器件
painter->setPen(QPen(Qt::black));
painter->drawRect(rect());
}
QPointF connectionPoint() const
{
// 返回连接点位置
return mapToScene(rect().center());
}
};
class ConnectionLine : public QGraphicsLineItem
{
public:
ConnectionLine(QGraphicsItem *startItem, QGraphicsItem *endItem, QGraphicsItem *parent = nullptr)
: QGraphicsLineItem(parent)
{
setPen(QPen(Qt::black, 2));
setLine(QLineF(startItem->scenePos(), endItem->scenePos()));
}
void updatePosition()
{
// 更新连接线位置
setLine(QLineF(startItem()->scenePos(), endItem()->scenePos()));
}
};
class CircuitScene : public QGraphicsScene
{
public:
CircuitScene(QObject *parent = nullptr)
: QGraphicsScene(parent)
{
}
void mousePressEvent(QGraphicsSceneMouseEvent *event) override
{
if (event->button() == Qt::LeftButton) {
// 创建新的器件
CircuitItem *item = new CircuitItem();
addItem(item);
item->setPos(event->scenePos());
// 记录拖拽起始位置
m_dragStartPos = event->scenePos();
// 记录当前拖拽的器件
m_draggingItem = item;
}
QGraphicsScene::mousePressEvent(event);
}
void mouseMoveEvent(QGraphicsSceneMouseEvent *event) override
{
if (m_draggingItem) {
// 判断是否需要进行复制操作
if ((event->scenePos() - m_dragStartPos).manhattanLength() > QApplication::startDragDistance()) {
// 创建新的器件
CircuitItem *item = new CircuitItem();
addItem(item);
item->setPos(m_draggingItem->pos() + event->scenePos() - m_dragStartPos);
// 记录当前拖拽的器件
m_draggingItem = item;
}
}
QGraphicsScene::mouseMoveEvent(event);
}
void mouseReleaseEvent(QGraphicsSceneMouseEvent *event) override
{
if (m_draggingItem) {
// 判断是否需要进行连接操作
for (QGraphicsItem *item : items(event->scenePos())) {
if (item != m_draggingItem && item->type() == CircuitItem::Type) {
CircuitItem *startItem = static_cast<CircuitItem *>(m_draggingItem);
CircuitItem *endItem = static_cast<CircuitItem *>(item);
// 创建新的连接线
ConnectionLine *line = new ConnectionLine(startItem, endItem);
addItem(line);
// 记录当前连接的器件
m_connectedItems.append(startItem);
m_connectedItems.append(endItem);
break;
}
}
// 清空当前拖拽的器件
m_draggingItem = nullptr;
}
QGraphicsScene::mouseReleaseEvent(event);
}
void drawBackground(QPainter *painter, const QRectF &rect) override
{
// 绘制背景
painter->setPen(QPen(Qt::lightGray));
for (int y = int(rect.top()); y <= int(rect.bottom()); ++y) {
for (int x = int(rect.left()); x <= int(rect.right()); ++x) {
if ((x + y) % 30 == 0) {
painter->drawPoint(x, y);
}
}
}
}
void drawForeground(QPainter *painter, const QRectF &rect) override
{
// 绘制连接线
painter->setPen(QPen(Qt::black, 2));
for (int i = 0; i < m_connectedItems.size(); i += 2) {
CircuitItem *startItem = m_connectedItems.at(i);
CircuitItem *endItem = m_connectedItems.at(i + 1);
painter->drawLine(startItem->connectionPoint(), endItem->connectionPoint());
}
}
private:
QPointF m_dragStartPos;
QGraphicsItem *m_draggingItem = nullptr;
QList<CircuitItem *> m_connectedItems;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QGraphicsView view;
view.setScene(new CircuitScene());
view.setRenderHint(QPainter::Antialiasing);
view.setDragMode(QGraphicsView::RubberBandDrag);
view.show();
return app.exec();
}
```
在这个示例中,我们创建了一个CircuitItem类作为器件的基类,继承自QGraphicsRectItem,并实现了paint函数来绘制器件。我们还创建了一个ConnectionLine类作为连接线的基类,继承自QGraphicsLineItem,并实现了updatePosition函数来更新连接线的位置。最后,我们创建了一个CircuitScene类作为场景的基类,继承自QGraphicsScene,并通过重载鼠标事件处理函数来实现器件的拖拽、复制和连接功能。在drawBackground和drawForeground函数中,我们还实现了背景和连接线的绘制功能。
阅读全文
相关推荐
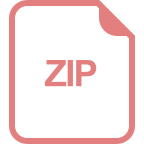
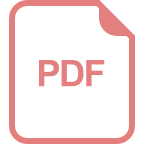








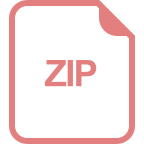