MATLAB图像配准算法代码
时间: 2024-11-07 20:11:30 浏览: 44
在MATLAB中,实现基本的图像配准通常涉及以下步骤,这里以使用`imregtform`函数为例,这是一个常用的仿射变换配准示例:
```matlab
% 加载图像
img1 = imread('image1.jpg');
img2 = imread('image2.jpg');
% 提取特征点,这里假设已经使用SIFT或SURF进行了特征检测
points1 = detectMinEigenFeatures(img1);
points2 = detectMinEigenFeatures(img2);
% 计算特征间的对应关系
[matches, ~] = matchFeatures(points1, points2, 'RatioTest', 0.8); % 使用RANSAC去除错误匹配
% 应用特征匹配到原始图像上,确定变换矩阵
tform = EstimateGeometricTransform(matches, 'affine');
% 将第二幅图像变换到第一幅图像的位置
warped_img2 = imwarp(img2, tform, 'OutputView', size(img1));
% 比较两幅图像,看配准是否成功
figure, imshowpair(img1, warped_img2, 'montage', 'border');
```
注意,这只是一个基本的示例,实际应用中可能需要考虑更多因素,如光照变化、噪声处理以及非线性变换(如透视变换)。如果你需要更高阶的配准方法,可以查阅MATLAB文档或研究论文了解其他高级技术。
相关问题
matlab图像配准代码
以下是一个基于互相关法的 MATLAB 图像配准代码示例:
```matlab
% 读取待配准的图像
fixed = imread('fixed_image.jpg');
moving = imread('moving_image.jpg');
% 将待配准图像转换为灰度图像
fixed_gray = rgb2gray(fixed);
moving_gray = rgb2gray(moving);
% 初始化配准参数
optimizer = registration.optimizer.OnePlusOneEvolutionary();
metric = registration.metric.MeanSquares();
[optimizer, metric] = imregconfig('multimodal');
optimizer.MaximumIterations = 1000;
% 使用互相关法进行图像配准
moving_reg = imregister(moving_gray, fixed_gray, 'affine', optimizer, metric);
% 显示配准结果
figure;
imshowpair(fixed_gray, moving_reg, 'montage');
title('配准结果');
```
上述代码中,首先使用 `imread` 函数读取待配准的图像,并使用 `rgb2gray` 函数将其转换为灰度图像。然后,使用 `imregconfig` 函数初始化配准参数,包括优化器和评估指标。接着,使用 `imregister` 函数进行图像配准,其中使用了互相关法和仿射变换模型。最后,使用 `imshowpair` 函数显示配准结果。
需要注意的是,图像配准是一个复杂的过程,不同的图像可能需要不同的配准算法和参数。此外,图像配准结果的质量也受到原始图像的质量和内容的影响。因此,对于特定的应用场景,可能需要对图像配准算法进行进一步的优化和调整。
图像配准算法matlab代码
以下是一个使用Matlab实现图像配准的简单案例代码:
```matlab
% 读取待配准的图像
fixedImage = imread('fixed_image.jpg');
movingImage = imread('moving_image.jpg');
% 将图像转换为灰度图像
fixedImageGray = rgb2gray(fixedImage);
movingImageGray = rgb2gray(movingImage);
% 提取图像特征点
fixedPoints = detectSURFFeatures(fixedImageGray);
movingPoints = detectSURFFeatures(movingImageGray);
% 提取特征描述子
[fixedFeatures, fixedPoints] = extractFeatures(fixedImageGray, fixedPoints);
[movingFeatures, movingPoints] = extractFeatures(movingImageGray, movingPoints);
% 匹配特征点
indexPairs = matchFeatures(fixedFeatures, movingFeatures);
% 选择匹配点对
fixedPoints = fixedPoints(indexPairs(:, 1));
movingPoints = movingPoints(indexPairs(:, 2));
% 估计图像变换矩阵
tform = estimateGeometricTransform(movingPoints, fixedPoints, 'affine');
% 对移动图像进行配准
registeredImage = imwarp(movingImage, tform, 'OutputView', imref2d(size(fixedImage)));
% 显示配准结果
figure;
imshowpair(fixedImage, registeredImage, 'montage');
title('配准结果');
% 保存配准结果
imwrite(registeredImage, 'registered_image.jpg');
```
这段代码实现了基于SURF特征的图像配准算法。首先,它读取待配准的图像,并将其转换为灰度图像。然后,它使用SURF算法提取图像的特征点和特征描述子。接下来,它通过匹配特征点找到匹配点对,并使用这些点对估计图像的变换矩阵。最后,它使用估计的变换矩阵对移动图像进行配准,并显示配准结果。
阅读全文
相关推荐
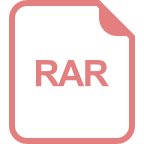
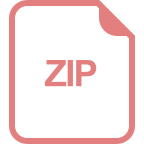
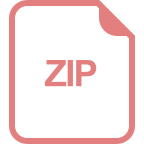
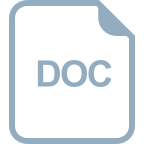
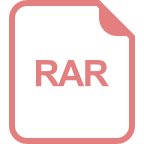
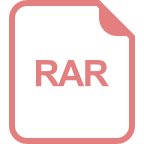
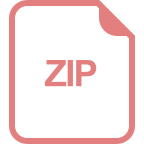


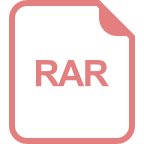
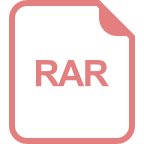
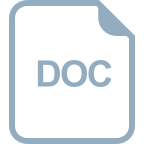
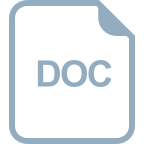
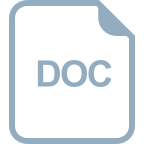



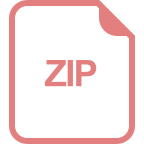