tf.nn.weighted_cross_entropy_with_logits(targets=y, logits=y_pred, pos_weight)函数的意思
时间: 2023-12-17 15:05:41 浏览: 31
`tf.nn.weighted_cross_entropy_with_logits`函数是用于计算加权的二分类交叉熵损失的函数。它的输入包括目标值(`targets`)、模型的预测值(`logits`)和正样本的权重(`pos_weight`)。
该函数的作用是计算二分类交叉熵损失,其中正样本的损失会乘以一个权重,可以用来调整正样本的重要性。正样本的权重越大,模型在预测时会更加关注正样本的正确分类。
具体而言,该函数首先将模型的预测值 `logits` 通过Sigmoid函数转换为概率值,然后将概率值与目标值 `targets` 计算交叉熵损失。在计算交叉熵损失时,正样本的损失会乘以 `pos_weight`。
使用示例:
```
loss = tf.nn.weighted_cross_entropy_with_logits(targets=y, logits=y_pred, pos_weight=pos_weight)
```
其中,`y` 是目标值(真实标签),`y_pred` 是模型的预测值(未经Sigmoid函数转换的logits),`pos_weight` 是正样本的权重。
需要注意的是,该函数适用于二分类问题,且要求 `logits` 的形状与 `targets` 相同。
相关问题
tf.nn.weighted_cross_entropy_with_logits
tf.nn.weighted_cross_entropy_with_logits是TensorFlow中的一个函数,用于计算加权交叉熵损失。它的输入是logits和labels,以及一个权重向量,用于加权不同类别的损失。该函数返回一个张量,表示加权交叉熵损失。
G=nx.Graph G.add_weighted_edges_from(edges)
这是一个Python中使用NetworkX库创建一个无向图G,并添加带权边的语句。其中,Graph()是NetworkX库中创建图的基本函数之一,用于创建一个空的图。G.add_weighted_edges_from(edges)则是使用NetworkX库中的函数将带权边添加到图G中。其中,edges应该是一个包含带权边信息的列表,可以使用类似 [(u, v, weight), (x, y, weight), ...] 的形式来表示。这个函数会将所有的带权边添加到图G中,如果节点不存在,则会自动添加该节点。
相关推荐
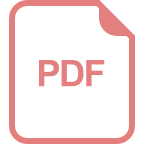












