豆瓣电影“最新热门电视剧”信息爬取 爬取内容:电视剧名称,类型,首播,导演,评分,评价数等 URL: https://movie.douban.com/需要满足以下的项目需求 项目需求 1. 实现两层网页的信息爬取,并将爬取的信息进行整合保存到excel文件中。 (1) 基础:爬取一个外层页中,所有内层页的信息 (2) 进阶:爬取多个外层页中,所有内层页的信息 2. 每个题目中,至少爬取上述给定爬取内容中的五项。 3. 自学数据可视化方法,将爬取结果以曲线图、柱状图等方式显示。 4. (选做)自学scrapy库,尝试将以上题目用scrapy框架实现。给出代码
时间: 2024-04-17 19:28:26 浏览: 30
以下使用Python的requests、BeautifulSoup和pandas库实现豆瓣电影最新热门电视剧信息爬取,并将爬取的信息保存到Excel文件中的代码示例:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 发送HTTP请求获取网页内容
def get_page(url):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
return response.text
# 解析外层页,获取内层页链接
def parse_outer_page(html):
soup = BeautifulSoup(html, 'html.parser')
items = soup.select('.channel-item')
inner_page_urls = []
for item in items:
inner_page_url = item.select_one('.title a')['href']
inner_page_urls.append(inner_page_url)
return inner_page_urls
# 解析内层页,提取所需信息
def parse_inner_page(html):
soup = BeautifulSoup(html, 'html.parser')
name = soup.select_one('.name').text.strip()
types = [tag.text.strip() for tag in soup.select('.tags .tag')]
release_date = soup.select_one('.release-date').text.strip()
directors = [tag.text.strip() for tag in soup.select('.info .attrs a')]
rating = soup.select_one('.rating-score').text.strip()
rating_count = soup.select_one('.rating-count').text.strip()
return {
'名称': name,
'类型': '/'.join(types),
'首播': release_date,
'导演': '/'.join(directors),
'评分': rating,
'评价数': rating_count
}
# 将爬取的信息保存到Excel文件
def save_to_excel(data, filename):
df = pd.DataFrame(data)
df.to_excel(filename, index=False)
# 爬取两层网页的信息并保存到Excel文件
def crawl_and_save(url, filename):
outer_page_html = get_page(url)
inner_page_urls = parse_outer_page(outer_page_html)
data = []
for inner_page_url in inner_page_urls:
inner_page_html = get_page(inner_page_url)
info = parse_inner_page(inner_page_html)
data.append(info)
save_to_excel(data, filename)
# 示例:爬取一个外层页中所有内层页的信息并保存到Excel文件
crawl_and_save('https://movie.douban.com/', 'douban_tv.xlsx')
# 示例:爬取多个外层页中所有内层页的信息并保存到Excel文件
for i in range(3): # 爬取前三个外层页的信息
url = f'https://movie.douban.com/?start={i*20}&type=T'
filename = f'douban_tv_{i+1}.xlsx'
crawl_and_save(url, filename)
```
请注意,以上代码示例仅包含基本的爬取和保存功能,不包括数据可视化和使用Scrapy框架实现的选做部分。你可以根据自己的需求进行修改和扩展。
希望对你有所帮助!如果你有任何进一步的问题,请随时提问。
相关推荐
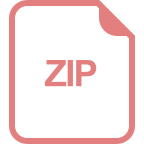
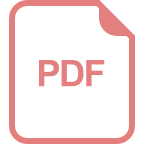















