SecretKeySpec secretKey = new SecretKeySpec(key.getBytes(), "AES"); try { Cipher cipher = Cipher.getInstance("AES"); cipher.init(1, secretKey); byte[] encodeBase64 = Base64.encodeBase64(content.getBytes("utf-8")); byte[] result = cipher.doFinal(encodeBase64); String hexString = Hex.encodeHexString(result); return hexString; } catch (Exception var7) { log.error("AES加密 - 异常", var7); return null; } 解释
时间: 2024-02-14 22:30:57 浏览: 79
这段代码是用于进行AES加密操作的。我会逐行解释它的功能:
1. 第一行创建了一个`SecretKeySpec`对象,用于存储密钥。其中`key.getBytes()`将密钥转换为字节数组,"AES"表示使用AES算法。
2. 接下来,使用`Cipher.getInstance("AES")`获取一个AES加密算法的实例。
3. `cipher.init(1, secretKey)`初始化Cipher对象,指定加密模式为1(加密模式),并传入密钥。
4. `Base64.encodeBase64(content.getBytes("utf-8"))`将待加密的内容转换为UTF-8编码的字节数组,并使用Base64进行编码。
5. `cipher.doFinal(encodeBase64)`对编码后的字节数组进行加密操作。
6. `Hex.encodeHexString(result)`将加密结果转换为十六进制字符串。
7. 最后,返回加密后的十六进制字符串。
如果在加密过程中出现异常,会记录错误日志并返回null。
相关问题
SecretKeySpec secretKey = new SecretKeySpec(key.getBytes(), "AES"); try { Cipher cipher = Cipher.getInstance("AES"); cipher.init(1, secretKey); byte[] encodeBase64 = Base64.encodeBase64(content.getBytes("utf-8")); byte[] result = cipher.doFinal(encodeBase64); String hexString = Hex.encodeHexString(result); return hexString; } catch (Exception var7) { log.error("AES加密 - 异常", var7); return null; } 如何进行解密
要进行解密,您需要相应的解密方法。下面是一个示例代码来演示如何使用AES解密:
```java
public static String decrypt(String encryptedText, String key) {
try {
SecretKeySpec secretKey = new SecretKeySpec(key.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] decodedHex = Hex.decodeHex(encryptedText.toCharArray());
byte[] decryptedBase64 = cipher.doFinal(decodedHex);
byte[] decodedBytes = Base64.decodeBase64(decryptedBase64);
return new String(decodedBytes, "UTF-8");
} catch (Exception e) {
log.error("AES解密 - 异常", e);
return null;
}
}
```
这段代码使用了与加密过程类似的步骤来进行解密:
1. 创建一个`SecretKeySpec`对象,用于存储密钥。
2. 获取一个AES加密算法的实例。
3. 初始化Cipher对象,指定解密模式(`Cipher.DECRYPT_MODE`)和密钥。
4. 将加密后的十六进制字符串转换为字节数组,使用`Hex.decodeHex()`方法。
5. 对字节数组进行解密操作,得到解密后的字节数组。
6. 对解密后的字节数组进行Base64解码,得到原始字节数组。
7. 使用UTF-8编码将字节数组转换为字符串,并返回解密结果。
如果在解密过程中出现异常,会记录错误日志并返回null。
SecretKey secretKey = new SecretKeySpec(sharedSecretA, "AES");
这行代码是用来创建一个 AES 对称加密算法的密钥对象 SecretKey 的。其中,sharedSecretA 是一个字节数组,通常是通过 Diffie-Hellman 密钥交换协议协商出来的共享密钥,用于双方进行加密通信。"AES" 则指定了使用 AES 算法进行加密。
创建 SecretKey 对象后,可以将其传递给 Cipher 对象,使用该对象进行加密或解密操作。例如:
```
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] encryptedData = cipher.doFinal(plainText.getBytes());
```
这段代码中,我们创建了一个 Cipher 对象,指定了使用 AES 算法和 CBC 模式进行加密,并且使用 PKCS5Padding 填充方式。然后,我们使用 init() 方法初始化 Cipher 对象,将 ENCRYPT_MODE 作为参数传入,表示我们要进行加密操作,同时将创建好的 SecretKey 对象传入,作为加密密钥。最后,我们使用 doFinal() 方法对明文进行加密操作,得到加密后的密文数据。
阅读全文
相关推荐
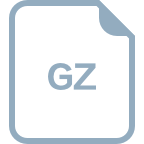
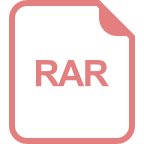
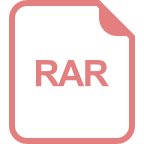













