浏览器开5个,配置文件分开。一直循环里面的定位元素
时间: 2024-04-18 15:29:12 浏览: 16
您可以使用多线程或多进程的方式来同时打开多个浏览器,并分别加载不同的配置文件。在每个线程或进程中,您可以使用浏览器自动化工具(如Selenium)来定位元素并执行操作。
以下是一个示例代码,使用Python的多线程和Selenium库来实现:
```python
import threading
from selenium import webdriver
# 定义一个函数,用于在每个线程中执行浏览器打开和定位元素的操作
def open_browser_and_locate_elements(config_file):
# 指定浏览器驱动的路径,这里以Chrome为例
driver_path = '/path/to/chromedriver'
# 使用指定的配置文件创建浏览器实例
options = webdriver.ChromeOptions()
options.add_argument('--user-data-dir=' + config_file)
driver = webdriver.Chrome(driver_path, options=options)
# 在这里执行定位元素并执行操作的代码
# 例如:
driver.get('https://www.example.com')
element = driver.find_element_by_id('element_id')
element.click()
# 关闭浏览器
driver.quit()
# 定义一个列表,存储配置文件路径
config_files = ['config1', 'config2', 'config3', 'config4', 'config5']
# 创建线程列表
threads = []
# 创建并启动线程
for config_file in config_files:
thread = threading.Thread(target=open_browser_and_locate_elements, args=(config_file,))
thread.start()
threads.append(thread)
# 等待所有线程执行完毕
for thread in threads:
thread.join()
```
在这个示例中,我们使用了五个配置文件(config1到config5),每个配置文件对应一个浏览器实例。每个线程会打开一个浏览器,并加载对应的配置文件。然后,您可以在`open_browser_and_locate_elements`函数中根据需求执行定位元素和操作的代码。最后,关闭浏览器。
注意:您需要根据您自己的环境和需求修改代码中的路径和操作。此外,根据您使用的浏览器和自动化工具,代码可能会有所不同。以上代码仅供参考。
相关推荐
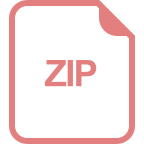
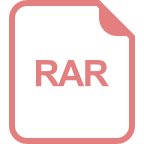
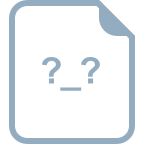














