你的回答中,如果将@Retention(RetentionPolicy.RUNTIME)修改为@Retention(RetentionPolicy.CLASS)代码和原来有什么区别?
时间: 2024-01-09 19:35:03 浏览: 89
如果我们将 `@Retention(RetentionPolicy.RUNTIME)` 修改为 `@Retention(RetentionPolicy.CLASS)`,代码的行为将发生以下变化:
1. 可见性:`@Retention(RetentionPolicy.CLASS)` 意味着注解在编译后的字节码中保留,但在运行时不可见。这意味着我们无法直接通过注解来执行特定的逻辑。只有通过反射等机制,在编译后的字节码中才能访问到注解。
2. 反射访问:由于注解在运行时不可见,我们需要使用反射机制来访问和处理这个注解。我们可以使用反射来获取类、方法或字段上的注解,并读取注解中的信息。通过反射,我们可以在运行时动态地获取和处理注解,执行相应的操作。
3. 编译期检查:与 `@Retention(RetentionPolicy.RUNTIME)` 不同,`@Retention(RetentionPolicy.CLASS)` 在编译期间就会检查注解是否被正确地使用。如果注解被错误地使用或应用到不正确的目标上(例如应用到类而不是方法上),编译器会报错。
总结起来,将 `@Retention(RetentionPolicy.RUNTIME)` 修改为 `@Retention(RetentionPolicy.CLASS)` 会导致注解在运行时不可见,只有在编译后的字节码中才能访问到它。我们需要通过反射来访问注解,并且在编译期间会进行注解的正确性检查。这样做是为了在编译时期进行更严格的控制和检查,以确保注解的正确使用。
相关问题
在你给我的这个回答的基础上,给我分别举@Retention(RetentionPolicy.CLASS)和@Retention(RetentionPolicy.RUNTIME)两个简单的例子
当一个自定义注解标记为 `@Retention(RetentionPolicy.CLASS)` 时,它会在编译后的字节码中保留,但在运行时不可见。我们可以通过反射来获取注解并执行一些操作。
下面是一个简单的示例:
```java
import java.lang.annotation.*;
@Retention(RetentionPolicy.CLASS)
@interface MyAnnotation {
String value();
}
public class MyClass {
@MyAnnotation("Hello")
public void myMethod() {
// do something
}
}
public class Main {
public static void main(String[] args) throws NoSuchMethodException {
MyClass obj = new MyClass();
// 使用反射获取方法上的注解
Method method = MyClass.class.getMethod("myMethod");
MyAnnotation annotation = method.getAnnotation(MyAnnotation.class);
if (annotation != null) {
String value = annotation.value();
System.out.println("Value: " + value); // 输出:Value: Hello
}
}
}
```
在这个例子中,`MyAnnotation` 自定义注解被标记为 `@Retention(RetentionPolicy.CLASS)`。我们通过反射获取 `myMethod` 方法上的注解,并输出注解中的值。
而当一个自定义注解标记为 `@Retention(RetentionPolicy.RUNTIME)` 时,它会在运行时保留,并可以通过反射来获取和处理。
下面是一个简单的示例:
```java
import java.lang.annotation.*;
@Retention(RetentionPolicy.RUNTIME)
@interface MyAnnotation {
String value();
}
public class MyClass {
@MyAnnotation("Hello")
public void myMethod() {
// do something
}
}
public class Main {
public static void main(String[] args) throws NoSuchMethodException {
MyClass obj = new MyClass();
// 使用反射获取方法上的注解
Method method = MyClass.class.getMethod("myMethod");
MyAnnotation annotation = method.getAnnotation(MyAnnotation.class);
if (annotation != null) {
String value = annotation.value();
System.out.println("Value: " + value); // 输出:Value: Hello
}
}
}
```
在这个例子中,`MyAnnotation` 自定义注解被标记为 `@Retention(RetentionPolicy.RUNTIME)`。我们同样通过反射获取 `myMethod` 方法上的注解,并输出注解中的值。
这两个例子中的注解都可以通过反射访问,并根据需要执行相应的操作。区别在于 `@Retention(RetentionPolicy.CLASS)` 注解在运行时不可见,只能在编译后的字节码中通过反射来访问,而 `@Retention(RetentionPolicy.RUNTIME)` 注解在运行时可见,可以直接通过反射来访问。
@Retention(RetentionPolicy.RUNTIME)
这是 Java 语言中的一个注解,用于指定被注解的元素的生命周期。具体来说,@Retention(RetentionPolicy.RUNTIME) 表示被注解的元素在运行时仍然可用,即在程序运行时可以通过反射等方式获取到该注解。这个注解通常用在自定义注解中,以便在运行时能够使用这些注解进行某些特定的操作或判断。
阅读全文
相关推荐
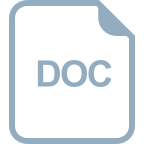
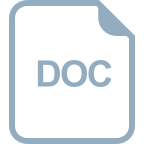
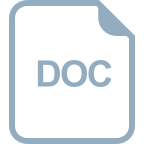













