利用c语言,对给定的循环链表,设计算法实现删除链表中所有重复的元素
时间: 2024-10-16 07:22:05 浏览: 7
在C语言中,可以使用哈希表(通常用数组或动态分配的数据结构实现)来辅助删除循环链表中重复的元素。下面是一种常见的解决方案:
1. 首先,我们需要定义一个结构体表示链表节点,并设置一个头结点。
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
2. 创建一个哈希表(这里假设使用数组)用于存储已经遇到的元素及其索引。
```c
#define TABLE_SIZE 100 // 假设哈希表大小足够大
int hash[TABLE_SIZE] = {0}; // 初始化全部为0
```
3. 定义一个辅助函数,用于查找元素是否已经在哈希表中。
```c
int isDuplicate(int key) {
return (hash[key % TABLE_SIZE] != 0);
}
```
4. 主函数中遍历链表,如果当前节点的值未在哈希表中,则添加到表中并将其指向下一个节点;如果已在表中,则将指针跳过已存在的节点。
```c
void removeDuplicates(Node* head) {
if (head == NULL || head->next == NULL)
return;
Node* current = head;
Node* temp = head->next;
while (temp != head) {
if (!isDuplicate(temp->data)) {
hash[temp->data % TABLE_SIZE] = 1; // 添加到哈希表
current->next = temp->next;
} else {
while (temp != head && !isDuplicate(temp->data))
temp = temp->next; // 跳过重复元素
current->next = temp->next;
}
current = current->next;
temp = current->next;
}
}
```
5. 最后,记得处理尾部的特殊情况,因为循环链表可能会包含自身作为重复元素。
```c
if (current->next == head)
current->next = NULL;
else
current->next = head;
```
阅读全文
相关推荐
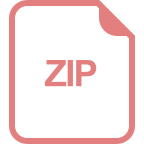
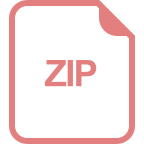
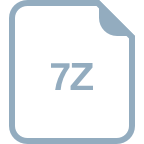















