人工神经网络c语言代码
时间: 2023-10-23 21:10:41 浏览: 83
好的,以下是一个简单的人工神经网络的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define INPUT_LAYER_SIZE 2
#define HIDDEN_LAYER_SIZE 3
#define OUTPUT_LAYER_SIZE 1
double sigmoid(double x) {
return 1.0 / (1.0 + exp(-x));
}
int main() {
double input[INPUT_LAYER_SIZE] = {1.0, 2.0};
double hidden[HIDDEN_LAYER_SIZE];
double output[OUTPUT_LAYER_SIZE];
double weights_ih[INPUT_LAYER_SIZE][HIDDEN_LAYER_SIZE] = {{0.1, 0.2, 0.3}, {0.4, 0.5, 0.6}};
double weights_ho[HIDDEN_LAYER_SIZE][OUTPUT_LAYER_SIZE] = {{0.7}, {0.8}, {0.9}};
// Forward pass
for (int i = 0; i < HIDDEN_LAYER_SIZE; i++) {
double sum = 0.0;
for (int j = 0; j < INPUT_LAYER_SIZE; j++) {
sum += input[j] * weights_ih[j][i];
}
hidden[i] = sigmoid(sum);
}
for (int i = 0; i < OUTPUT_LAYER_SIZE; i++) {
double sum = 0.0;
for (int j = 0; j < HIDDEN_LAYER_SIZE; j++) {
sum += hidden[j] * weights_ho[j][i];
}
output[i] = sigmoid(sum);
}
printf("Output: %f\n", output[0]);
return 0;
}
```
这个代码实现了一个简单的前向传播的过程,其中包括一个输入层、一个隐藏层和一个输出层。输入层有两个神经元,隐藏层有三个神经元,输出层有一个神经元。权重矩阵 `weights_ih` 和 `weights_ho` 分别表示输入层到隐藏层和隐藏层到输出层的权重。
相关推荐
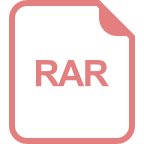
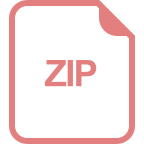
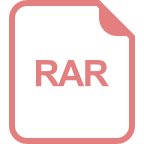














