将上述python代码转化为C语言代码
时间: 2024-10-07 12:08:21 浏览: 37
将上述Python代码转换为C语言代码,你需要使用字符串处理功能和条件判断来代替Python中的类似特性。以下是一个简单的示例,展示了如何使用C语言实现相似的功能,包括词法分析和结果输出:
```c
#include <stdio.h>
#include <ctype.h>
#include <stdbool.h>
#include <string.h>
#define MAX_WORD_LENGTH 30
typedef struct {
int code;
const char* symbol;
} Word;
Word parse_word(const char* source, int* cursor) {
Word word;
memset(&word, 0, sizeof(Word));
word.code = 0;
while (*cursor < strlen(source)) {
if (isalnum(*source)) {
word.symbol = realloc(word.symbol, (strlen(word.symbol) + 1) * sizeof(char));
word.symbol[strlen(word.symbol)] = *source;
++(*cursor);
} else if (*source == ',') {
word.code = get_separator_code(",");
break;
} else {
// 添加对其他分隔符和关键字、标识符、常数、运算符处理的逻辑
word.code = get_special_character_code(*source);
break;
}
}
return word;
}
void output_words(const Word* words, size_t count) {
for (size_t i = 0; i < count; ++i) {
printf("(%d, \"%s\")\n", words[i].code, words[i].symbol);
}
}
// ... 在这里定义函数get_keyword_id(), get_identifier_id(), get_constant_value(), get_operator_code(), get_special_character_code() 等
int main() {
const char* source_code = "main() {int a=-5,b=4,j; if(a>=b) j=a-b; else j=b-a;}";
int cursor = 0;
Word words[MAX_WORDS];
size_t word_count = 0;
// 词法分析
while (cursor < strlen(source_code)) {
Word parsed_word = parse_word(&source_code[cursor], &cursor);
words[word_count++] = parsed_word;
}
// 输出结果
output_words(words, word_count);
return 0;
}
```
在这个C代码中,我们假设已经实现了`isalnum()`、`get_separator_code()`, `get_keyword_id()`, `get_identifier_id()`, `get_constant_value()`, `get_operator_code()` 和 `get_special_character_code()` 函数,用于识别各类单词并返回对应的编码。注意,C语言没有内置的动态内存分配,所以字符串处理稍微复杂一些,需要你自己管理字符串长度和内存分配。
阅读全文
相关推荐
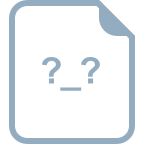
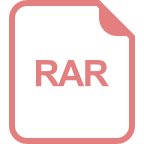
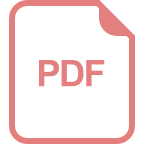













