tostring c++
时间: 2024-12-24 18:15:52 浏览: 3
在C++中,`to_string()`并不是一个标准库函数,但如果你正在使用C++11及以上版本,你可以使用内置的`std::to_string()`函数将其他数据类型转换为字符串。这个函数属于头文件`<sstream>`,可以用于整数、浮点数、字符等基本类型的转换。例如:
```cpp
int num = 42;
std::string strNum = std::to_string(num);
```
如果你想自定义一个对象的转换为字符串,可以覆盖默认的`operator <<`重载到`std::ostream&`,类似于这样:
```cpp
class MyClass {
public:
// 重载to_string函数
friend std::ostream& operator<<(std::ostream& os, const MyClass& obj) {
return os << "MyClass object: " << obj.someMember;
}
};
MyClass myObj;
std::string strMyObj = myObj; // 这里会自动调用to_string重载
```
相关问题
c++tostring
As an AI language model, I am assuming you are referring to the `toString()` method which is commonly used in programming languages to convert an object to a string representation.
In JavaScript, the `toString()` method can be used on any object to get a string representation of that object. For example:
```
let num = 123;
let str = num.toString();
console.log(str); // Output: "123"
```
In Java, the `toString()` method is used to get a string representation of an object. It is a method of the `Object` class, and can be overridden in subclasses to provide a customized string representation of the object. For example:
```
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
}
// Example usage:
Person person = new Person("John", 30);
String str = person.toString();
System.out.println(str); // Output: Person{name='John', age=30}
```
C++tostring
C++中没有内置的`tostring`函数,但是可以通过自定义函数或者使用库来实现类似的功能。`tostring`函数通常用于将一个对象转换为字符串表示形式,以便于输出或者进行其他操作。
在C++中,可以通过重载`<<`运算符来实现对象的输出。例如,如果有一个名为`Person`的类,可以在类中定义一个友元函数`operator<<`,并在函数中将对象的属性输出到流中。这样,在使用`cout`输出对象时,就会调用该函数。
以下是一个简单的示例:
```cpp
#include <iostream>
#include <string>
class Person {
private:
std::string name;
int age;
public:
Person(const std::string& n, int a) : name(n), age(a) {}
friend std::ostream& operator<<(std::ostream& os, const Person& person) {
os << "Name: " << person.name << ", Age: " << person.age;
return os;
}
};
int main() {
Person p("Alice", 25);
std::cout << p << std::endl; // 输出:Name: Alice, Age: 25
return 0;
}
```
在上述示例中,我们重载了`<<`运算符,并定义了一个友元函数`operator<<`,该函数将对象的属性输出到流中。
阅读全文
相关推荐
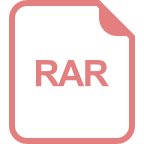
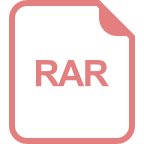
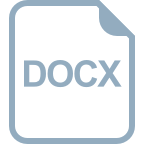













