c语言模拟excel表格的排序
时间: 2023-12-09 12:35:44 浏览: 183
以下是使用C语言模拟Excel表格排序的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_ROW 1000
#define MAX_COL 100
// 定义学生结构体
typedef struct {
char id[20];
char name[20];
int score;
} Student;
// 定义全局变量
Student students[MAX_ROW];
int row_num = 0;
int col_num = 3;
// 比较函数,用于qsort排序
int cmp(const void *a, const void *b) {
Student *s1 = (Student *)a;
Student *s2 = (Student *)b;
if (s1->score != s2->score) {
return s1->score - s2->score;
} else if (strcmp(s1->name, s2->name) != 0) {
return strcmp(s1->name, s2->name);
} else {
return strcmp(s1->id, s2->id);
}
}
// 读取数据
void read_data() {
FILE *fp = fopen("data.txt", "r");
if (fp == NULL) {
printf("Failed to open file!\n");
exit(1);
}
char buf[1024];
while (fgets(buf, 1024, fp) != NULL) {
char *p = strtok(buf, ",");
strcpy(students[row_num].id, p);
p = strtok(NULL, ",");
strcpy(students[row_num].name, p);
p = strtok(NULL, ",");
students[row_num].score = atoi(p);
row_num++;
}
fclose(fp);
}
// 输出数据
void print_data() {
for (int i = 0; i < row_num; i++) {
printf("%s,%s,%d\n", students[i].id, students[i].name, students[i].score);
}
}
// 排序并输出数据
void sort_data(int c) {
qsort(students, row_num, sizeof(Student), cmp);
if (c == 1) {
for (int i = 0; i < row_num; i++) {
printf("%s,%s,%d\n", students[i].id, students[i].name, students[i].score);
}
} else if (c == 2) {
for (int i = 0; i < row_num; i++) {
printf("%s,%s,%d\n", students[i].id, students[i].name, students[i].score);
}
} else if (c == 3) {
for (int i = 0; i < row_num; i++) {
printf("%s,%s,%d\n", students[i].id, students[i].name, students[i].score);
}
} else {
printf("Invalid parameter!\n");
}
}
int main() {
read_data();
printf("Original data:\n");
print_data();
printf("Sorted data:\n");
sort_data(2);
return 0;
}
```
该示例代码中,我们定义了一个`Student`结构体,用于存储学生的信息,包括学号、姓名和成绩。我们使用`read_data()`函数从文件中读取数据,并使用`print_data()`函数输出原始数据。然后,我们使用`sort_data()`函数对数据进行排序,并输出排序后的结果。在`sort_data()`函数中,我们使用`qsort()`函数对学生数组进行排序,排序的依据是题目中所给的要求。最后,我们根据参数`c`的值,选择不同的排序方式输出结果。
阅读全文
相关推荐
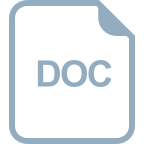
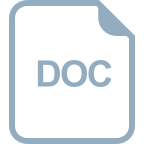
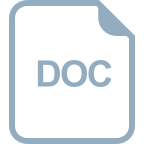
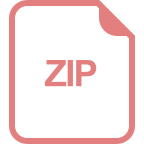
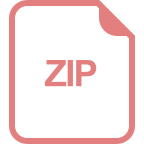
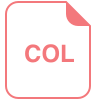
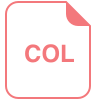
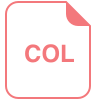
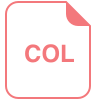
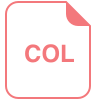
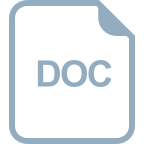
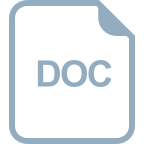
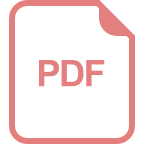
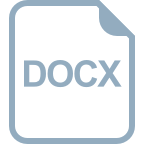
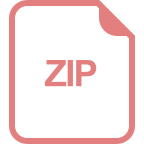
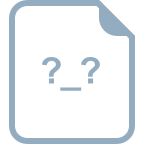
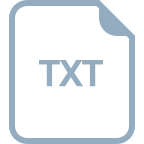