HAL_UART_Transmit_DMA 发送字符串
时间: 2024-01-28 20:13:39 浏览: 31
```c
#include "stm32f4xx_hal.h"
UART_HandleTypeDef huart;
void HAL_UART_Transmit_DMA_String(UART_HandleTypeDef *huart, char *str)
{
// 等待串口空闲
while (huart->gState == HAL_UART_STATE_BUSY_TX)
{
// 可以添加延时函数,等待上一条数据发送完成
}
// 发送字符串
HAL_UART_Transmit_DMA(huart, (uint8_t *)str, strlen(str));
}
```
相关问题
HAL_UART_Transmit_DMA 发送字符串数组
以下是使用HAL_UART_Transmit_DMA发送字符串数组的示例代码:
```c
#include "stm32l1xx_hal.h"
#define UART_BUFFER_SIZE 100
UART_HandleTypeDef huart;
void HAL_UART_TxCpltCallback(UART_HandleTypeDef *huart)
{
// UART transmit complete callback function
// This function is called when the DMA transfer is complete
// You can perform any necessary operations here
}
void HAL_UART_Transmit_DMA_StringArray(uint8_t *strArray[], uint16_t arraySize)
{
// Calculate the total length of the string array
uint16_t totalLength = 0;
for (uint16_t i = 0; i < arraySize; i++)
{
totalLength += strlen((char *)strArray[i]);
}
// Create a buffer to hold the concatenated string
uint8_t buffer[UART_BUFFER_SIZE];
memset(buffer, 0, UART_BUFFER_SIZE);
// Concatenate the strings into the buffer
uint16_t bufferIndex = 0;
for (uint16_t i = 0; i < arraySize; i++)
{
strcpy((char *)(buffer + bufferIndex), (char *)strArray[i]);
bufferIndex += strlen((char *)strArray[i]);
}
// Start the DMA transfer
HAL_UART_Transmit_DMA(&huart, buffer, totalLength);
}
```
使用上述代码,你可以通过调用`HAL_UART_Transmit_DMA_StringArray`函数来发送一个字符串数组。该函数会将字符串数组中的所有字符串连接起来,并使用DMA进行传输。
HAL_UART_Transmit_DMA 发送char 数组
可以使用HAL_UART_Transmit_DMA函数来发送char数组。该函数的参数包括UART_HandleTypeDef结构体指针、pData指向要发送的数据的指针、Size表示要发送的数据的大小以及Timeout表示发送超时时间。下面是一个示例代码:
```c
char data[] = "Hello, world!";
uint16_t size = sizeof(data) - 1; // 减去字符串末尾的空字符
HAL_UART_Transmit_DMA(&huart, (uint8_t*)data, size);
```
在上面的代码中,我们定义了一个char数组data,并初始化为"Hello, world!"。然后,我们使用sizeof运算符获取数组的大小,并减去1,以去除末尾的空字符。最后,我们调用HAL_UART_Transmit_DMA函数来发送char数组。
请注意,上述代码中的huart是UART_HandleTypeDef类型的结构体指针,需要根据实际情况进行修改。
相关推荐
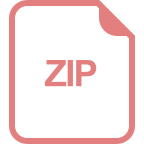











