class GraphPooling(nn.Module): def __init__(self, pool_type): super(GraphPooling, self).__init__() self.pool_type = pool_type if pool_type == 'mean': self.reduce_func = fn.mean(msg='m', out='h') elif pool_type == 'max': self.reduce_func = fn.max(msg='m', out='h') elif pool_type == 'min': self.reduce_func = fn.min(msg='m', out='h') def forward(self, g, feat): with g.local_scope(): g.ndata['x'] = feat g.update_all(fn.copy_u('x', 'm'), self.reduce_func) return g.ndata['h']
时间: 2024-04-18 19:27:51 浏览: 110
这段代码定义了一个名为GraphPooling的神经网络模块,用于对图进行池化操作。
在初始化方法__init__中,通过传入参数pool_type来指定池化操作的类型。如果pool_type为'mean',则使用fn.mean函数进行平均池化;如果pool_type为'max',则使用fn.max函数进行最大池化;如果pool_type为'min',则使用fn.min函数进行最小池化。
在forward方法中,输入参数g表示输入的图,feat表示节点的特征表示。在每次前向传播过程中,首先将特征表示feat赋值给图g中的节点数据字段'x'。然后使用g.update_all函数,根据消息传递规则fn.copy_u('x', 'm')将节点特征'x'复制到边上的消息'm'中,并使用预定义的reduce_func对消息进行池化操作。最后,返回经过池化操作后的节点特征'h'。
这个GraphPooling模块可以方便地根据不同的池化类型对输入的图进行池化操作,并提取出整体图的特征表示。
相关问题
class Model(nn.Module): def __init__(self): super().__init__() self.conv1 = nn.Conv2d(1, 20, 5)
self.pool = nn.MaxPool2d(2, 2) self.conv2 = nn.Conv2d(20, 50, 5) self.fc1 = nn.Linear(4*4*50, 500) self.fc2 = nn.Linear(500, 10) def forward(self, x): x = self.pool(F.relu(self.conv1(x))) x = self.pool(F.relu(self.conv2(x))) x = x.view(-1, 4*4*50) x = F.relu(self.fc1(x)) x = self.fc2(x) return x
This is a PyTorch model for classifying handwritten digits using a convolutional neural network. The model consists of two convolutional layers followed by two fully connected layers. The first convolutional layer has 20 filters of size 5x5 and takes in a grayscale image of size 28x28. The second convolutional layer has 50 filters of size 5x5. The output of the convolutional layers is passed through a max pooling layer with a kernel size of 2x2. The output of the max pooling layer is then flattened and passed through two fully connected layers with ReLU activation. The final output is a probability distribution over the 10 possible digit classes.
class LeNet(nn.Module): def __init__(self): super(LeNet, self).__init__()
这是一个使用PyTorch实现的LeNet模型的定义,LeNet是一个经典的卷积神经网络模型,用于图像分类任务。
在这个代码中,LeNet继承自nn.Module,表示LeNet是一个PyTorch的模型,nn.Module是PyTorch中所有神经网络模型的基类。
__init__方法是LeNet的构造函数,用于初始化模型中的各个组件(如卷积层、池化层、全连接层等),并将它们组合在一起形成整个模型。在这个代码中,super(LeNet, self).__init__()调用了父类nn.Module的构造函数,初始化模型。
在__init__方法中,我们需要定义模型中的各个层,这些层可以通过nn.Module中提供的各种层来定义。例如,可以使用nn.Conv2d来定义卷积层,使用nn.MaxPool2d来定义池化层,使用nn.Linear来定义全连接层等。具体的实现可以参考LeNet的论文或者其他实现代码。
阅读全文
相关推荐
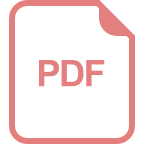
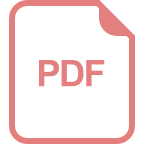
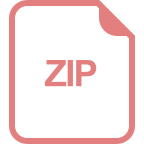












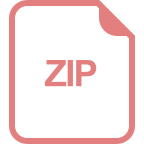