class h_sigmoid(nn.Module): def __init__(self, inplace=True): super(h_sigmoid, self).__init__() self.relu = nn.ReLU6(inplace=inplace) def forward(self, x): return self.relu(x + 3) / 6 class h_swish(nn.Module): def __init__(self, inplace=True): super(h_swish, self).__init__() self.sigmoid = h_sigmoid(inplace=inplace) def forward(self, x): return x * self.sigmoid(x) class CoordAtt(nn.Module): def __init__(self, inp, oup, reduction=32): super(CoordAtt, self).__init__() # height方向上的均值池化 self.pool_h = nn.AdaptiveAvgPool2d((None, 1)) # width方向上的均值池化 self.pool_w = nn.AdaptiveAvgPool2d((1, None)) mip = max(8, inp // reduction) self.conv1 = nn.Conv2d(inp, mip, kernel_size=1, stride=1, padding=0) self.bn1 = nn.BatchNorm2d(mip) self.act = h_swish() self.conv_h = nn.Conv2d(mip, oup, kernel_size=1, stride=1, padding=0) self.conv_w = nn.Conv2d(mip, oup, kernel_size=1, stride=1, padding=0) def forward(self, x): identity = x n, c, h, w = x.size() x_h = self.pool_h(x) x_w = self.pool_w(x).permute(0, 1, 3, 2) y = torch.cat([x_h, x_w], dim=2) y = self.conv1(y) y = self.bn1(y) y = self.act(y) x_h, x_w = torch.split(y, [h, w], dim=2) x_w = x_w.permute(0, 1, 3, 2) a_h = self.conv_h(x_h).sigmoid() a_w = self.conv_w(x_w).sigmoid() out = identity * a_w * a_h return out 嵌入CA注意力机制后出现这个问题怎么解决TypeError: init() takes from 3 to 4 positional arguments but 5 were given
时间: 2024-01-02 10:03:08 浏览: 170
根据您提供的代码,我看到在CoordAtt类的初始化函数中,没有发现任何与错误消息匹配的问题。根据错误提示,init()函数接受3到4个位置参数,但是在您的代码中没有传递额外的参数。
因此,可能的原因是您在其他地方调用了CoordAtt类的初始化函数,并传递了额外的参数。请检查一下您的代码,确保在初始化CoordAtt类时没有传递多余的参数。
另外,如果您在其他地方使用了CoordAtt类的实例,并且传递了额外的参数,那么可能需要修改相应的代码,以确保与CoordAtt类的初始化函数匹配。
如果问题仍然存在,请提供更多的代码和上下文信息,这样我可以更好地帮助您解决问题。
相关问题
class SelfAttention(nn.Module): def init(self, in_channels, reduction=4): super(SelfAttention, self).init() self.avg_pool = nn.AdaptiveAvgPool1d(1) self.fc1 = nn.Conv1d(in_channels, in_channels // reduction, 1, bias=False) self.relu = nn.ReLU(inplace=True) self.fc2 = nn.Conv1d(in_channels // reduction, in_channels, 1, bias=False) self.sigmoid = nn.Sigmoid() def forward(self, x): b, c, n = x.size() y = self.avg_pool(x) y = self.fc1(y) y = self.relu(y) y = self.fc2(y) y = self.sigmoid(y) return x * y.expand_as(x) def get_model(input_channels=6, use_xyz=True): return Pointnet2MSG(input_channels=input_channels, use_xyz=use_xyz) class Pointnet2MSG(nn.Module): def init(self, input_channels=6, use_xyz=True): super().init() self.SA_modules = nn.ModuleList() channel_in = input_channels skip_channel_list = [input_channels] for k in range(cfg.RPN.SA_CONFIG.NPOINTS.len()): mlps = cfg.RPN.SA_CONFIG.MLPS[k].copy() channel_out = 0 for idx in range(mlps.len()): mlps[idx] = [channel_in] + mlps[idx] channel_out += mlps[idx][-1] mlps.append(channel_out) self.SA_modules.append( nn.Sequential( PointnetSAModuleMSG( npoint=cfg.RPN.SA_CONFIG.NPOINTS[k], radii=cfg.RPN.SA_CONFIG.RADIUS[k], nsamples=cfg.RPN.SA_CONFIG.NSAMPLE[k], mlps=mlps, use_xyz=use_xyz, bn=cfg.RPN.USE_BN ), SelfAttention(channel_out) ) ) skip_channel_list.append(channel_out) channel_in = channel_out self.FP_modules = nn.ModuleList() for k in range(cfg.RPN.FP_MLPS.len()): pre_channel = cfg.RPN.FP_MLPS[k + 1][-1] if k + 1 < len(cfg.RPN.FP_MLPS) else channel_out self.FP_modules.append( PointnetFPModule( mlp=[pre_channel + skip_channel_list[k]] + cfg.RPN.FP_MLPS[k] ) )根据如上代码,如果要在Pointnet2MSG类中的forward函数调用SA_modules的话需要传入哪些参数,几个参数?
在Pointnet2MSG类中的forward函数调用SA_modules时,需要传入点云数据x以及额外的参数,具体如下:
```python
def forward(self, x):
l_xyz, l_features = [None] * self.num_layers, [None] * self.num_layers
# SA_modules
for i in range(self.num_layers):
# SA_modules需要传入的参数包括:输入点云数据x,输入点云xyz坐标l_xyz[i-1],输入点云特征向量l_features[i-1]
xyz, features = self.SA_modules[i][0](x if i == 0 else l_xyz[i-1], l_features[i-1])
l_xyz[i], l_features[i] = xyz, features
# SelfAttention模块需要传入的参数:输入特征向量l_features[i]
l_features[i] = self.SA_modules[i][1](l_features[i])
# FP_modules
for i in range(self.num_layers-1, -1, -1):
# FP_modules需要传入的参数包括:上一层的输出xyz坐标l_xyz[i+1],上一层的输出特征向量l_features[i+1],当前层的输入xyz坐标l_xyz[i],当前层的输入特征向量l_features[i]
l_features[i] = self.FP_modules[i](l_xyz[i+1], l_xyz[i], l_features[i+1], l_features[i])
return l_features[0]
```
其中,SA_modules需要传入的参数包括:输入点云数据x,输入点云xyz坐标l_xyz[i-1],输入点云特征向量l_features[i-1],SelfAttention模块需要传入的参数为输入特征向量l_features[i]。FP_modules需要传入的参数包括:上一层的输出xyz坐标l_xyz[i+1],上一层的输出特征向量l_features[i+1],当前层的输入xyz坐标l_xyz[i],当前层的输入特征向量l_features[i]。
class DropBlock_Ske(nn.Module): def __init__(self, num_point, block_size=7): super(DropBlock_Ske, self).__init__() self.keep_prob = 0.0 self.block_size = block_size self.num_point = num_point self.fc_1 = nn.Sequential( nn.Linear(in_features=25, out_features=25, bias=True), nn.ReLU(inplace=True), nn.Linear(in_features=25, out_features=25, bias=True), ) self.fc_2 = nn.Sequential( nn.Linear(in_features=25, out_features=25, bias=True), nn.ReLU(inplace=True), nn.Linear(in_features=25, out_features=25, bias=True), ) self.sigmoid = nn.Sigmoid() def forward(self, input, keep_prob, A): # n,c,t,v self.keep_prob = keep_prob if not self.training or self.keep_prob == 1: return input n, c, t, v = input.size() input_attention_mean = torch.mean(torch.mean(input, dim=2), dim=1).detach() # 32 25 input_attention_max = torch.max(input, dim=2)[0].detach() input_attention_max = torch.max(input_attention_max, dim=1)[0] # 32 25 avg_out = self.fc_1(input_attention_mean) max_out = self.fc_2(input_attention_max) out = avg_out + max_out input_attention_out = self.sigmoid(out).view(n, 1, 1, self.num_point) input_a = input * input_attention_out input_abs = torch.mean(torch.mean( torch.abs(input_a), dim=2), dim=1).detach() input_abs = input_abs / torch.sum(input_abs) * input_abs.numel() gamma = 0.024 M_seed = torch.bernoulli(torch.clamp( input_abs * gamma, min=0, max=1.0)).to(device=input.device, dtype=input.dtype) M = torch.matmul(M_seed, A) M[M > 0.001] = 1.0 M[M < 0.5] = 0.0 mask = (1 - M).view(n, 1, 1, self.num_point) return input * mask * mask.numel() / mask.sum()
这段代码定义了一个名为DropBlock_Ske的PyTorch模型类。该类继承自nn.Module基类,并实现了模型的前向传播逻辑。
在初始化方法中,定义了一些模型的属性,包括keep_prob、block_size、num_point等。然后,定义了两个全连接网络层fc_1和fc_2,并使用nn.Sequential组织网络层的结构。最后,使用nn.Sigmoid定义了一个Sigmoid激活函数。
在前向传播方法中,首先根据输入的keep_prob值判断是否需要执行DropBlock操作,如果不需要则直接返回输入。接着,获取输入张量的形状,并计算出输入张量的均值和最大值。通过两个全连接网络层对均值和最大值进行处理,将处理结果相加得到out。然后,使用Sigmoid激活函数对out进行处理,得到一个形状为(n, 1, 1, num_point)的张量input_attention_out。将input_attention_out与输入张量input相乘得到input_a。
接下来,计算input_a的绝对值的平均值,并将其除以总数并乘以元素个数,得到一个形状为(n,)的张量input_abs。定义了一个gamma值,并将input_abs与gamma相乘并经过torch.clamp函数进行限制,再经过torch.bernoulli函数进行伯努利采样,得到一个形状与输入相同的二值张量M_seed。使用torch.matmul函数将M_seed与A矩阵相乘得到M。
然后,将M中大于0.001的元素赋值为1.0,小于0.5的元素赋值为0.0。接着,将1减去M得到mask,将mask乘以输入张量input,并除以mask中的元素个数与总和,得到最终的输出张量。
这个模型类实现了DropBlock_Ske操作,其中包含了一些全连接网络层和一些基于概率的操作。它的具体功能和用途可能需要根据上下文来确定。
阅读全文
相关推荐
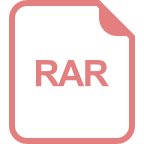
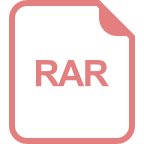








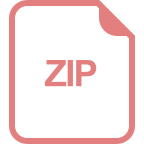