vim Pingpong.c hadoop00@ubuntu:~/exp1$ gcc Pingpong.c -o Pingpong hadoop00@ubuntu:~/exp1$ ./Pingpong a Result from child: a 修改代码
时间: 2024-11-30 21:15:54 浏览: 7
在给定的环境中,你已经编译了一个名为`Pingpong.c`的C程序,并运行了它。在这个程序中,我们看到的是一个简单的管道示例,其中父进程从用户那里读取一个字符,将其传递给子进程,子进程将其加一后返回给父进程。代码大概如下:
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
// 假设这是你的Pingpong.c内容
int main() {
int pipe_fd[2];
char input[1], output[1];
if (pipe(pipe_fd) != 0) {
perror("Failed to create pipe");
return 1;
}
close(pipe_fd[1]); // 父进程负责读
// 从用户输入读取字符
input[0] = getchar();
printf("Received input: %c\n", input[0]);
// 写入管道
if (write(pipe_fd[0], input, sizeof(input)) != sizeof(input)) {
perror("Write to pipe failed");
return 1;
}
// 创建子进程并等待
pid_t pid = fork();
if (pid < 0) {
perror("Fork failed");
return 1;
} else if (pid == 0) { // 子进程
close(pipe_fd[0]);
input[0]++;
if (write(pipe_fd[1], input, sizeof(input)) != sizeof(input)) {
perror("Write back to pipe failed");
_exit(1);
}
_exit(0); // 子进程结束
}
// 父进程读回结果
close(pipe_fd[0]);
read(pipe_fd[1], output, sizeof(output));
printf("Result from child: %c\n", output[0]);
return 0;
}
```
如果你想要修改代码,这里有一些可能的方向:
1. **增加错误检查**:可以在关键点添加更多的错误检查,比如检查输入、读写操作是否成功等。
2. **用户交互**:可以询问用户是否要继续循环或输入其他字符,直到用户选择停止。
3. **使用字符串**:如果需要处理更复杂的数据,可以考虑使用字符串而不是单个字符。
4. **改进并发处理**:如果你计划处理多个请求,可以使用非阻塞I/O或线程池来提高性能。
如果你想进一步修改,请提供具体的更改需求,我会帮助你进行相应的调整。
阅读全文
相关推荐
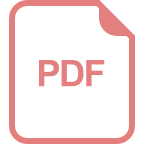
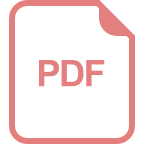
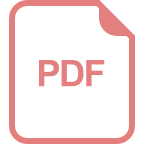




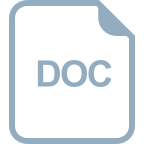



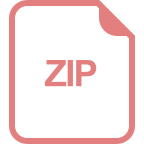





