sftp登录可以if判断么
时间: 2024-09-15 11:07:37 浏览: 32
SFTP(Secure File Transfer Protocol)是一种安全的文件传输协议,通常用于远程登录并操作服务器上的文件。在 Bash 脚本中,你可以使用 `if` 来进行条件判断,比如检查 SFTP 连接是否成功。以下是一个基本的例子:
```bash
#!/bin/bash
sftp_user="username"
sftp_host="hostname"
sftp_dir="directory"
# 使用 ssh 连接到 SFTP 服务器
sftp -o ConnectTimeout=5 "$sftp_user@$sftp_host" << EOF
cd "$sftp_dir"
# 假设你想检查当前目录是否为空,如果为空则执行后续操作
if ls | wc -l == 0; then
echo "目录 $sftp_dir 是空的"
# 接下来的命令如果连接成功且目录为空,则执行
else
echo "目录 $sftp_dir 非空"
fi
exit
EOF
# 如果连接失败,后续的 if 语句不会被执行
# ... (这里可以添加处理失败情况的代码)
相关问题
sftp判断文件是否存在
在SFTP(SSH File Transfer Protocol,安全Shell 文件传输协议)中检查文件是否存在的操作通常涉及到SSH客户端库的应用程序。在Python等支持SFTP的编程语言里,你可以利用`paramiko`这样的库来进行这类操作。
首先,你需要安装并导入`paramiko`库。如果你还没有这个库,可以使用pip命令进行安装:
```bash
pip install paramiko
```
然后,你可以通过下面的步骤检查远程服务器上特定文件的存在情况:
```python
import paramiko
def check_file_exists(host, username, password, remote_path):
# 创建SSH连接
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
try:
ssh.connect(host, username=username, password=password)
# 使用SFTP创建通道
sftp = ssh.open_sftp()
try:
# 尝试打开文件获取其属性
sftp.stat(remote_path)
print(f"文件 {remote_path} 存在")
return True
except FileNotFoundError:
print(f"文件 {remote_path} 不存在")
return False
finally:
# 关闭资源
sftp.close()
ssh.close()
# 需要替换为你实际的信息
host = 'your_server_ip'
username = 'your_username'
password = 'your_password'
remote_path = '/path/to/your/file'
if check_file_exists(host, username, password, remote_path):
pass
else:
pass
```
### 相关问题:
1. `check_file_exists()` 函数是如何工作的?
2. 如果需要同时检查多个文件怎么办?
3. 这种方法对网络延迟敏感吗?
java,sftp判断路径是否存在
你可以使用JSch库来实现SFTP协议的文件操作,包括路径判断。具体步骤如下:
1. 连接SFTP服务器,获取SFTP会话对象。
```java
JSch jsch = new JSch();
Session session = jsch.getSession(username, host, port);
session.setPassword(password);
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
ChannelSftp sftp = (ChannelSftp) session.openChannel("sftp");
sftp.connect();
```
2. 判断路径是否存在。
```java
try {
sftp.cd(path);
return true;
} catch (SftpException e) {
if (e.id == ChannelSftp.SSH_FX_NO_SUCH_FILE) {
return false;
}
throw new RuntimeException(e);
}
```
其中,`sftp.cd(path)` 会尝试切换到指定路径,如果路径不存在则会抛出 `SftpException` 异常,异常的 `id` 值为 `ChannelSftp.SSH_FX_NO_SUCH_FILE`,表示文件不存在。
完整代码示例:
```java
import com.jcraft.jsch.ChannelSftp;
import com.jcraft.jsch.JSch;
import com.jcraft.jsch.Session;
import com.jcraft.jsch.SftpException;
public class SftpDemo {
public static void main(String[] args) {
String host = "sftp.example.com";
int port = 22;
String username = "username";
String password = "password";
String path = "/path/to/directory";
JSch jsch = new JSch();
try {
Session session = jsch.getSession(username, host, port);
session.setPassword(password);
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
ChannelSftp sftp = (ChannelSftp) session.openChannel("sftp");
sftp.connect();
boolean exists = existsDirectory(sftp, path);
System.out.println("Path exists: " + exists);
sftp.disconnect();
session.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
public static boolean existsDirectory(ChannelSftp sftp, String path) {
try {
sftp.cd(path);
return true;
} catch (SftpException e) {
if (e.id == ChannelSftp.SSH_FX_NO_SUCH_FILE) {
return false;
}
throw new RuntimeException(e);
}
}
}
```
阅读全文
相关推荐
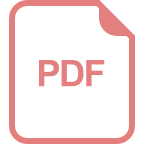
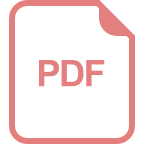
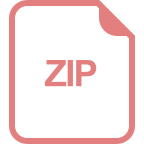
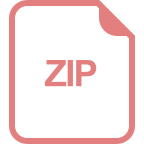








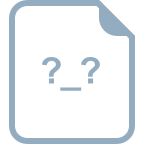
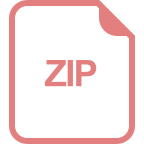
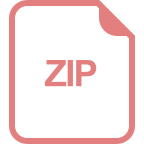