(1)从键盘输入几名学生的姓名和年龄,(假设学生姓名中都没有空格)以二进制文件形式存储该程序,生成一个学生记录文件 students.dat。 (2)编程实现将(1)创建的学生记录文件 students.dat 的内容读出并显示。资源环境为visual c++
时间: 2024-12-04 15:24:07 浏览: 15
要实现这个功能,你需要分两步进行:
1. **编写程序保存学生信息到二进制文件**
首先,我们需要从键盘获取学生的姓名和年龄,然后将其保存到二进制文件`students.dat`。这里使用`ofstream`来操作二进制文件。
```cpp
#include <iostream>
#include <fstream>
using namespace std;
struct Student {
string name;
int age;
};
void saveStudentsToFile() {
int numStudents;
cout << "Enter the number of students: ";
cin >> numStudents;
ofstream outFile("students.dat", ios::binary);
if (!outFile) {
cerr << "Error opening file!" << endl;
return;
}
for (int i = 0; i < numStudents; ++i) {
Student student;
cout << "Enter student name and age for student " << (i + 1) << ": ";
cin >> student.name >> student.age;
// 将Student对象转换为字节流并写入文件
char* byteData = reinterpret_cast<char*>(&student);
outFile.write(byteData, sizeof(Student));
}
outFile.close();
cout << "Students saved to students.dat" << endl;
}
// 第二步的代码
```
2. **编程读取并显示文件中的学生信息**
然后,我们可以编写一个函数来从文件中读取这些数据并显示出来。这需要用到`ifstream`。
```cpp
void readStudentsFromFile() {
ifstream inFile("students.dat", ios::binary);
if (!inFile) {
cerr << "Error opening file!" << endl;
return;
}
while (true) {
Student student;
inFile.read(reinterpret_cast<char*>(&student), sizeof(Student));
if (inFile.eof()) break; // 文件已读完
cout << "Name: " << student.name << ", Age: " << student.age << endl;
}
inFile.close();
cout << "Student records read from students.dat" << endl;
}
int main() {
saveStudentsToFile();
readStudentsFromFile();
return 0;
}
```
**相关问题--:**
1. 如何使用`ios::binary`标志打开文件?
2. `reinterpret_cast<char*>()`的作用是什么?
3. `eof()`函数是如何检测文件末尾的?
4. 为什么在读取文件后关闭文件?
阅读全文
相关推荐
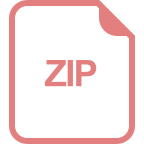
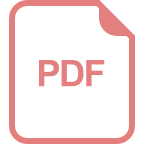
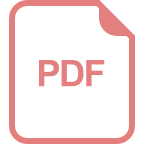

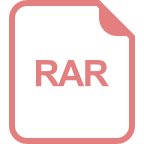
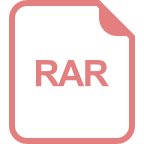
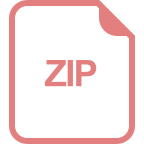
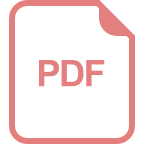
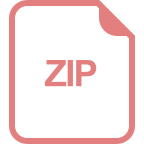
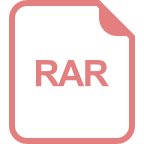
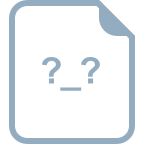
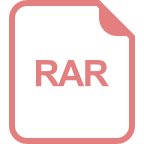
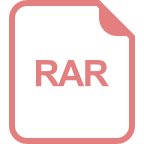
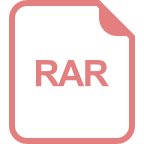
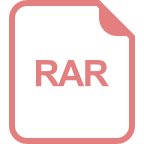
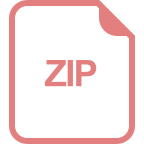
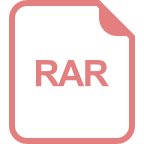
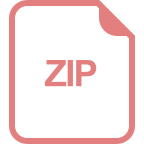
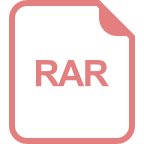