nn.Sequential( Transpose((0, 3, 1, 2)), layer_init(nn.Conv2d(2, 16, kernel_size=3, stride=2)), nn.SiLU(), layer_init(nn.Conv2d(16, 32, kernel_size=2)), nn.SiLU(), layer_init(nn.Conv2d(32, 32, kernel_size=2)), nn.SiLU(), layer_init(nn.Conv2d(32, 32, kernel_size=2)), nn.SiLU(), layer_init(nn.Conv2d(32, 32, kernel_size=2)), nn.SiLU(), nn.Flatten(), layer_init(nn.Linear(32 * 9 * 9, 128)), nn.SiLU(), )
时间: 2024-04-14 20:30:00 浏览: 85
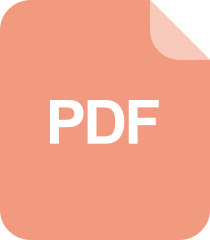
对Pytorch中nn.ModuleList 和 nn.Sequential详解
这是一个用于图像处理的神经网络模型,它包含了一系列的卷积神经网络层和激活函数。具体的结构如下:
1. Transpose:将输入的维度进行转置,将通道维度放到第二个位置。
2. nn.Conv2d:卷积层,输入通道数为2,输出通道数为16,卷积核大小为3x3,步长为2。
3. nn.SiLU:激活函数,使用SiLU(即Sigmoid-Weighted Linear Unit)。
4. nn.Conv2d:卷积层,输入通道数为16,输出通道数为32,卷积核大小为2x2。
5. nn.SiLU:激活函数,使用SiLU。
6. nn.Conv2d:卷积层,输入通道数为32,输出通道数为32,卷积核大小为2x2。
7. nn.SiLU:激活函数,使用SiLU。
8. nn.Conv2d:卷积层,输入通道数为32,输出通道数为32,卷积核大小为2x2。
9. nn.SiLU:激活函数,使用SiLU。
10. nn.Conv2d:卷积层,输入通道数为32,输出通道数为32,卷积核大小为2x2。
11. nn.SiLU:激活函数,使用SiLU。
12. nn.Conv2d:卷积层,输入通道数为32,输出通道数为32,卷积核大小为2x2。
13. nn.SiLU:激活函数,使用SiLU。
14. nn.Flatten:将多维的输入展平为一维。
15. nn.Linear:全连接层,输入大小为32x9x9,输出大小为128。
16. nn.SiLU:激活函数,使用SiLU。
这个模型的输入是一个大小为HxWx2的张量(H和W是图像的高度和宽度),输出是一个大小为128的向量。
阅读全文
相关推荐
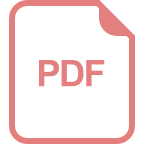
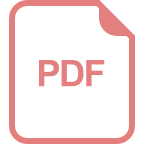















