在vue3中实现父子组件通信,用自定义事件来解决,用代码实现,详细一点
时间: 2024-04-16 17:23:50 浏览: 18
当在 Vue.js 3 中需要实现父子组件之间的通信时,可以使用自定义事件来解决。下面是一个详细的代码示例:
父组件 `ParentComponent.vue`:
```vue
<template>
<div>
<h2>父组件</h2>
<button @click="sendMessageToChild">发送消息给子组件</button>
<ChildComponent @custom-event="handleCustomEvent"></ChildComponent>
</div>
</template>
<script>
import { ref } from 'vue';
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
methods: {
sendMessageToChild() {
this.$refs.childComponent.$emit('custom-event', 'Hello from parent!'); // 发送自定义事件给子组件
},
handleCustomEvent(message) {
console.log('父组件接收到子组件的消息:', message);
}
}
}
</script>
```
子组件 `ChildComponent.vue`:
```vue
<template>
<div>
<h3>子组件</h3>
</div>
</template>
<script>
export default {
methods: {
handleButtonClick() {
this.$emit('custom-event', 'Hello from child!'); // 触发自定义事件,并传递消息给父组件
}
}
}
</script>
```
在上述示例中,父组件通过 `@click` 监听按钮的点击事件,并调用 `sendMessageToChild` 方法来发送消息给子组件。在子组件中,我们通过 `this.$emit` 触发了一个名为 `custom-event` 的自定义事件,并将消息作为参数传递给父组件。
在父组件中,我们通过 `@custom-event` 监听子组件触发的 `custom-event` 事件,并在 `handleCustomEvent` 方法中接收到子组件发送的消息。
希望这个示例能够帮助你理解在 Vue.js 3 中使用自定义事件实现父子组件通信的方式。如果还有其他问题,请随时提问!
相关推荐
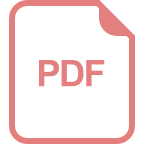
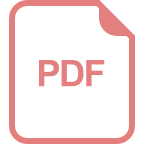
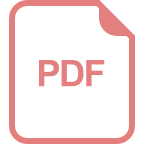
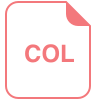
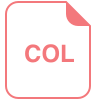
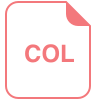
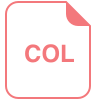
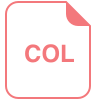









